#include <stdio.h> #include <stdlib.h> #include <time.h> // 判断是否为闰年 int is_leap_year(int year) { return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0; } // 计算某年某月的天数 int get_days_in_month(int year, int month) { int days[] = {31, 28 + is_leap_year(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; return days[month - 1]; } // 计算某年某月第一天是星期几 int get_weekday(int year, int month) { struct tm time = {0}; time.tm_year = year - 1900; time.tm_mon = month - 1; time.tm_mday = 1; mktime(&time); return time.tm_wday; } // 显示日历 void show_calendar(int year, int month) { int days = get_days_in_month(year, month); int weekday = get_weekday(year, month); printf("%d年%d月\n", year, month); printf("日 一 二 三 四 五 六\n"); for (int i = 0; i < weekday; i++) { printf(" "); } for (int i = 1; i <= days; i++) { printf("%2d ", i); if ((i + weekday) % 7 == 0) { printf("\n"); } } printf("\n"); } int main() { time_t now = time(NULL); struct tm *tm_now = localtime(&now); int year = tm_now->tm_year + 1900; int month = tm_now->tm_mon + 1; show_calendar(year, month); while (1) { printf("请输入年份和月份(例如:2022 1):"); scanf("%d %d", &year, &month); if (year < 1900 || year > 9999 || month < 1 || month > 12) { printf("输入错误,请重新输入!\n"); continue; } show_calendar(year, month); } return 0; }
时间: 2024-04-27 17:22:36 浏览: 74
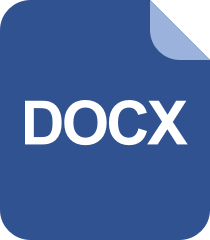
哈尔滨工业大学C语言实验题.docx
这是一段 C 语言代码,实现了一个简单的日历程序。它包含了判断是否为闰年、计算某年某月的天数、计算某年某月第一天是星期几、以及显示日历等功能。程序会先显示当前年月的日历,然后等待用户输入年份和月份,显示对应月份的日历。值得注意的是,程序使用了 C 标准库中的 time.h 和 stdlib.h 头文件,以及 struct tm 结构体和 mktime() 函数等库函数,这些函数可以方便地进行时间的处理和转换。
阅读全文
相关推荐
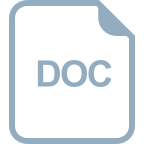
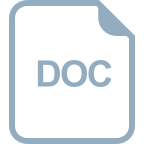















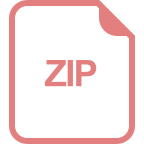