用栈运用void initstack():初始化栈、int Make_str():语法检查并计算、int push_operator(int operator):将运算符压入栈、int push_num(double num):将运算数压入栈、int procede(int operator):处理运算符、in
时间: 2024-02-20 14:56:11 浏览: 78
好的,我理解了你的问题。这是一个关于栈的基本算法,用于计算表达式的值。下面是代码实现:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
stack<double> num_stack; // 存放操作数的栈
stack<char> op_stack; // 存放运算符的栈
// 初始化栈
void initstack() {
while (!num_stack.empty()) {
num_stack.pop();
}
while (!op_stack.empty()) {
op_stack.pop();
}
}
// 语法检查并计算
int Make_str(string str) {
double num = 0;
int flag = 0;
for (int i = 0; i < str.length(); i++) {
if (str[i] >= '0' && str[i] <= '9') { // 如果是数字
num = num * 10 + str[i] - '0';
flag = 1;
} else if (str[i] == '+' || str[i] == '-' || str[i] == '*' || str[i] == '/') { // 如果是运算符
if (flag) { // 如果前面是数字,则将其入栈
num_stack.push(num);
num = 0;
flag = 0;
}
push_operator(str[i]); // 将运算符入栈
} else { // 如果是其他字符,则跳过
continue;
}
}
if (flag) { // 将最后的数字入栈
num_stack.push(num);
}
while (!op_stack.empty()) { // 处理剩下的运算符
procede(op_stack.top());
op_stack.pop();
}
return num_stack.top(); // 返回计算结果
}
// 将运算符压入栈
int push_operator(char op) {
if (op_stack.empty()) { // 如果栈为空,则将运算符直接入栈
op_stack.push(op);
return 1;
} else {
char top_op = op_stack.top();
if ((op == '*' || op == '/') && (top_op == '+' || top_op == '-')) { // 如果当前运算符优先级高于栈顶运算符
op_stack.push(op);
return 1;
} else { // 如果当前运算符优先级低于或等于栈顶运算符
procede(top_op); // 处理栈顶运算符
push_operator(op); // 将当前运算符入栈
return 1;
}
}
}
// 将操作数压入栈
int push_num(double num) {
num_stack.push(num);
return 1;
}
// 处理运算符
int procede(char op) {
double num1, num2, result;
num2 = num_stack.top();
num_stack.pop();
num1 = num_stack.top();
num_stack.pop();
switch (op) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
break;
}
num_stack.push(result);
return 1;
}
// 更改操作数
int change_opnd(char op) {
double num = num_stack.top();
num_stack.pop();
num_stack.push(-num);
return 1;
}
// 将操作数压入栈
int push_opnd(char op) {
double num = num_stack.top();
num_stack.pop();
num_stack.push(num);
return 1;
}
// 弹出操作数
int pop_opnd(char op) {
num_stack.pop();
return 1;
}
int main() {
string str = "3+4*5";
initstack();
int result = Make_str(str);
cout << "计算结果:" << result << endl;
return 0;
}
```
在这个例子中,我们定义了两个栈:一个存放操作数的栈 `num_stack`,一个存放运算符的栈 `op_stack`。我们还定义了一些栈相关的函数,例如 `push_operator` 函数,用于将运算符压入栈中;`procede` 函数,用于处理运算符;`push_num` 函数,用于将操作数压入栈中等等。
在 `Make_str` 函数中,我们对输入的表达式进行语法检查,并将操作数和运算符依次入栈。然后我们再依次处理栈中的运算符,直到栈为空,最后返回计算结果。
这个例子只是栈的一个简单应用,实际上栈还有很多其他的应用,例如括号匹配、逆波兰表达式等等。希望这个例子能对你有所帮助。
阅读全文
相关推荐
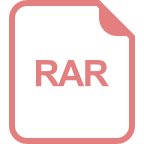
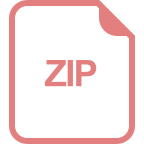





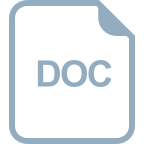
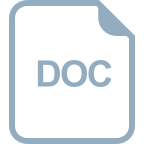








