uct { ElemType data[MaxSize]; //存放共享栈中元素 int top1,top2; //两个栈的栈顶指针 } DStack; 共享栈类型: 初始化InitStack(S) 入栈Push(S,i,x) 出栈Pop(S,i,x) 注:其中i为0或1 ,用以表示栈号。 作业1:共享栈 请为此双向栈设计完成以下功能定
时间: 2023-05-19 14:06:31 浏览: 112
义:
1. 初始化共享栈
2. 判断共享栈是否为空
3. 判断共享栈是否已满
4. 入栈操作
5. 出栈操作
6. 获取共享栈中某个位置的元素值
7. 修改共享栈中某个位置的元素值
以下是共享栈的实现代码:
#define MaxSize 100
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];
int top1, top2;
} DStack;
// 初始化共享栈
void InitStack(DStack *S) {
S->top1 = -1;
S->top2 = MaxSize;
}
// 判断共享栈是否为空
int IsEmpty(DStack *S, int i) {
if (i == 0) {
return S->top1 == -1;
} else {
return S->top2 == MaxSize;
}
}
// 判断共享栈是否已满
int IsFull(DStack *S) {
return S->top1 + 1 == S->top2;
}
// 入栈操作
int Push(DStack *S, int i, ElemType x) {
if (IsFull(S)) {
return 0;
}
if (i == 0) {
S->data[++S->top1] = x;
} else {
S->data[--S->top2] = x;
}
return 1;
}
// 出栈操作
int Pop(DStack *S, int i, ElemType *x) {
if (IsEmpty(S, i)) {
return 0;
}
if (i == 0) {
*x = S->data[S->top1--];
} else {
*x = S->data[S->top2++];
}
return 1;
}
// 获取共享栈中某个位置的元素值
int GetElem(DStack *S, int i, int pos, ElemType *x) {
if (i == 0) {
if (pos < 0 || pos > S->top1) {
return 0;
}
*x = S->data[pos];
} else {
if (pos < S->top2 || pos >= MaxSize) {
return 0;
}
*x = S->data[pos];
}
return 1;
}
// 修改共享栈中某个位置的元素值
int SetElem(DStack *S, int i, int pos, ElemType x) {
if (i == 0) {
if (pos < 0 || pos > S->top1) {
return 0;
}
S->data[pos] = x;
} else {
if (pos < S->top2 || pos >= MaxSize) {
return 0;
}
S->data[pos] = x;
}
return 1;
}
阅读全文
相关推荐
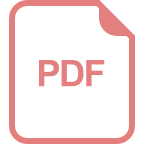
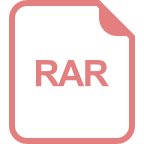
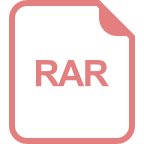
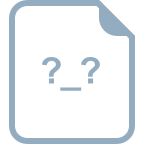
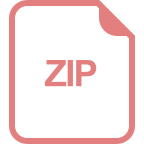
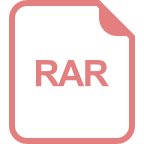
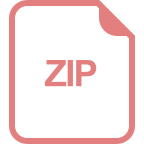
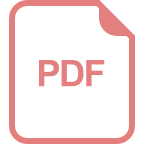
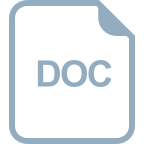
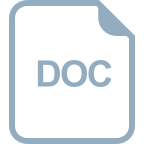
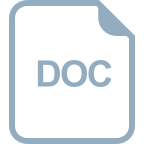
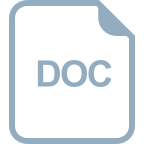





