python雪花id 实现
时间: 2023-06-29 15:04:40 浏览: 153
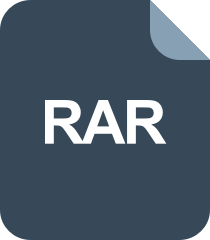
多语言版16位、18位、19位雪花ID总汇,包含Java、Go、Python、NodeJs、C#(含.Net core),PHP、Rust、SQL版。
雪花ID是一种分布式唯一ID生成算法,它可以在分布式系统中生成全局唯一的ID,通常用于分布式系统中的数据标识和分布式锁等场景。下面是一个简单的Python实现:
```python
import time
class SnowflakeID:
def __init__(self, datacenter_id, worker_id):
self.datacenter_id = datacenter_id
self.worker_id = worker_id
self.sequence = 0
self.last_timestamp = -1
self.twepoch = 1288834974657
def next_id(self):
timestamp = int(time.time() * 1000)
if timestamp < self.last_timestamp:
raise ValueError("Clock moved backwards. Refusing to generate id")
if timestamp == self.last_timestamp:
self.sequence = (self.sequence + 1) & 4095
if self.sequence == 0:
timestamp = self.wait_for_next_millis(self.last_timestamp)
else:
self.sequence = 0
self.last_timestamp = timestamp
return ((timestamp - self.twepoch) << 22) | (self.datacenter_id << 17) | (self.worker_id << 12) | self.sequence
def wait_for_next_millis(self, last_timestamp):
timestamp = int(time.time() * 1000)
while timestamp <= last_timestamp:
timestamp = int(time.time() * 1000)
return timestamp
```
使用示例:
```python
id_generator = SnowflakeID(datacenter_id=1, worker_id=1)
id = id_generator.next_id()
print(id)
```
在这个实现中,我们把SnowflakeID类实例化时传入的`datacenter_id`和`worker_id`用于生成ID。`sequence`是一个12位的计数器,用于在同一毫秒内生成不同的ID。`last_timestamp`是上一次生成ID的时间戳,如果当前时间戳小于上一次的时间戳,说明时钟回拨了,此时抛出异常。同时,如果当前时间戳与上一次的时间戳相同,需要通过自旋等待下一个毫秒的到来。最后,我们将`timestamp`、`datacenter_id`、`worker_id`和`sequence`按照一定的规则组合成一个64位的整数作为ID返回。
阅读全文
相关推荐
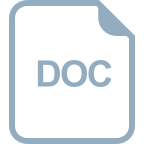
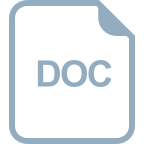
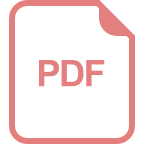
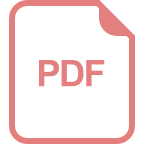



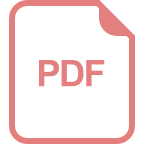
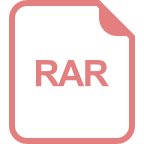
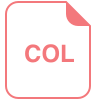







