1.有A和B两个数组,A数组中存储500个2000以内的随机整数,B数组中存储10个2000以内的随机整数,完成以下操作: (1)用顺序查找算法显示B数组中每个元素在A数组中的位置,并返回该算法所用时间; (2)用折半查找算法显示B数组中每个元素在A数组中的位置,并返回该算法所用时间; 部分参考代码如下: #include<ctime> //使用函数库ctime …… int main() { clock_t start,finish; //定义查找开始时间和结束时间变量 …… start=clock(); //记录查找算法的开始时间 查找算法 finish=clock(); //记录查找算法的结束时间 …… }
时间: 2024-03-25 13:37:34 浏览: 43
好的,针对您的问题,我可以给出以下代码实现:
```cpp
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
const int N = 500;
const int M = 10;
// 顺序查找算法
int sequential_search(int array[], int len, int target) {
for (int i = 0; i < len; i++) {
if (array[i] == target) {
return i;
}
}
return -1;
}
// 折半查找算法
int binary_search(int array[], int len, int target) {
int left = 0, right = len - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (array[mid] == target) {
return mid;
}
else if (array[mid] < target) {
left = mid + 1;
}
else {
right = mid - 1;
}
}
return -1;
}
int main() {
int A[N], B[M];
// 生成随机数
srand((unsigned)time(NULL));
for (int i = 0; i < N; i++) {
A[i] = rand() % 2000 + 1;
}
for (int i = 0; i < M; i++) {
B[i] = rand() % 2000 + 1;
}
// 顺序查找
clock_t start_seq = clock();
for (int i = 0; i < M; i++) {
int index = sequential_search(A, N, B[i]);
cout << "B[" << i << "]在A中的位置为:" << index << endl;
}
clock_t end_seq = clock();
// 折半查找
clock_t start_bin = clock();
for (int i = 0; i < M; i++) {
int index = binary_search(A, N, B[i]);
cout << "B[" << i << "]在A中的位置为:" << index << endl;
}
clock_t end_bin = clock();
// 输出时间
cout << "顺序查找算法用时:" << (double)(end_seq - start_seq) / CLOCKS_PER_SEC << "秒" << endl;
cout << "折半查找算法用时:" << (double)(end_bin - start_bin) / CLOCKS_PER_SEC << "秒" << endl;
return 0;
}
```
在上面的代码中,首先定义了顺序查找算法和折半查找算法的函数,然后生成了两个数组A和B,分别存储500个和10个随机整数。接着,通过调用 `clock()` 函数获取时间戳,记录顺序查找和折半查找的开始时间和结束时间,并计算出两个算法的用时。最后,输出每个元素在A数组中的位置和两个算法的用时。
需要注意的是,这里的时间单位是秒,可以通过 `CLOCKS_PER_SEC` 来将时间戳转换成秒数。同时,为了保证每次生成的随机数不同,我们需要在程序开始时调用 `srand()` 函数设置随机数种子。
阅读全文
相关推荐
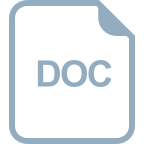
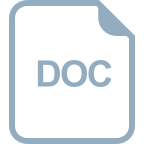
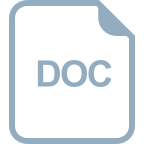






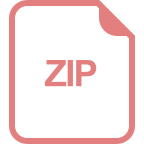

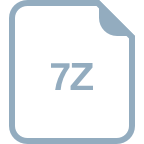
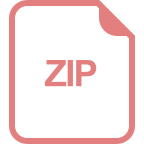
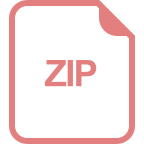
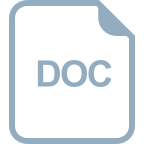



