以时钟类为例,将运算符++和--(包括前置和后置)重载为时钟类的成员函数,操作 数是时钟类的对象,实现时间增加(或减少)1 秒钟。时钟类的声明如下,要求实现 类;写出主函数,创建时钟类对象,使用重载运算符。
时间: 2024-02-15 15:02:58 浏览: 151
好的,以下是时钟类的声明和重载运算符的实现:
```c++
#include<iostream>
using namespace std;
class Clock {
public:
Clock(int h, int m, int s);
void showTime();
Clock& operator ++(); // 前置++
Clock operator ++(int); // 后置++
Clock& operator --(); // 前置--
Clock operator --(int); // 后置--
private:
int hour, minute, second;
};
Clock::Clock(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
void Clock::showTime() {
cout << hour << ":" << minute << ":" << second << endl;
}
Clock& Clock::operator ++() {
second++;
if (second >= 60) {
second = 0;
minute++;
if (minute >= 60) {
minute = 0;
hour++;
if (hour >= 24) {
hour = 0;
}
}
}
return *this;
}
Clock Clock::operator ++(int) {
Clock old = *this;
++(*this);
return old;
}
Clock& Clock::operator --() {
second--;
if (second < 0) {
second = 59;
minute--;
if (minute < 0) {
minute = 59;
hour--;
if (hour < 0) {
hour = 23;
}
}
}
return *this;
}
Clock Clock::operator --(int) {
Clock old = *this;
--(*this);
return old;
}
int main() {
Clock c(10, 30, 0);
c.showTime();
++c;
c.showTime();
c++;
c.showTime();
--c;
c.showTime();
c--;
c.showTime();
return 0;
}
```
在主函数中创建了一个时钟对象 `c`,并使用重载运算符 `++` 和 `--` 实现了时间增加和减少。需要注意的是,前置和后置的重载函数返回值类型不同,前置返回引用,后置返回对象。
阅读全文
相关推荐
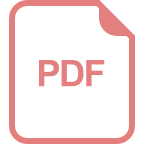


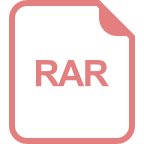
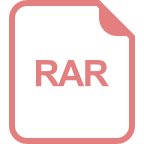
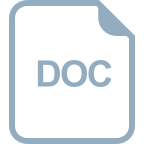
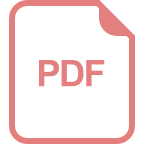
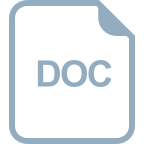
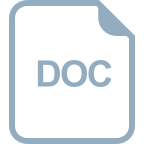


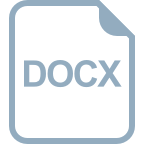