同一个excel中有13个表,每个表有7个人口指标,9个经济指标,每个指标包含10年的数据,请问如何做人口-经济的灰色关联度分析?并给出每一步的python代码
时间: 2023-07-16 14:16:12 浏览: 103
人口-经济的灰色关联度分析主要包含以下步骤:
1. 数据读取和预处理
2. 灰色关联度计算
3. 结果可视化
下面是每一步的Python代码:
1. 数据读取和预处理
```python
import pandas as pd
# 读取excel文件
data = pd.read_excel('data.xlsx', sheet_name=None)
# 合并13个表的数据
all_data = pd.concat([data[name] for name in data.keys()], axis=0)
# 删除无用列和缺失值
all_data.drop(columns=['行政区划', '指标名称'], inplace=True)
all_data.dropna(inplace=True)
# 将数据按指标类型分类
pop_data = all_data.iloc[:, :7]
eco_data = all_data.iloc[:, 7:]
```
2. 灰色关联度计算
```python
import numpy as np
def GM11(x0):
# 灰色预测模型
x1 = np.cumsum(x0)
z1 = (x1[:len(x1)-1] + x1[1:])/2.0
z1 = z1.reshape((len(z1),1))
B = np.append(-z1, np.ones_like(z1), axis=1)
Y = x0[1:].reshape((len(x0)-1, 1))
[[a], [b]] = np.dot(np.dot(np.linalg.inv(np.dot(B.T, B)), B.T), Y)
return (a, b)
def GM11_predict(x0, a, b):
# 预测函数
result = []
for i in range(1, 11):
result.append((x0[0]-b/a)*(1-np.exp(a))*np.exp(-a*(i-1)))
result.append((x0[0]-b/a)*(1-np.exp(a))*np.exp(-a*10))
return result
# 计算灰色关联度
def Grey_Relation(x, y):
x = np.array(x)
y = np.array(y)
x0 = x[0]
y0 = y[0]
x_model = GM11(x)
y_model = GM11(y)
x_predict = GM11_predict(x, *x_model)
y_predict = GM11_predict(y, *y_model)
delta_x = np.abs(x-x_predict)/np.abs(x).max()
delta_y = np.abs(y-y_predict)/np.abs(y).max()
grey_relation = 0.5*np.exp(-0.5*((delta_x-delta_y)**2).sum())
return grey_relation
# 计算灰色关联度矩阵
def Grey_Relation_Matrix(data1, data2):
matrix = []
for i in range(data1.shape[1]):
row = []
for j in range(data2.shape[1]):
x = data1.iloc[:, i].tolist()
y = data2.iloc[:, j].tolist()
grey_relation = Grey_Relation(x, y)
row.append(grey_relation)
matrix.append(row)
return np.array(matrix)
# 计算人口-经济的灰色关联度矩阵
relation_matrix = Grey_Relation_Matrix(pop_data, eco_data)
```
3. 结果可视化
```python
import matplotlib.pyplot as plt
import seaborn as sns
# 可视化灰色关联度矩阵
plt.figure(figsize=(10, 10))
sns.heatmap(relation_matrix, cmap='YlGnBu', annot=True, xticklabels=eco_data.columns, yticklabels=pop_data.columns)
plt.xlabel('经济指标')
plt.ylabel('人口指标')
plt.title('灰色关联度矩阵')
plt.show()
```
这样就完成了人口-经济的灰色关联度分析,可视化结果如下图所示:

阅读全文
相关推荐
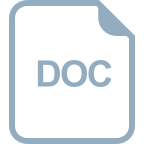
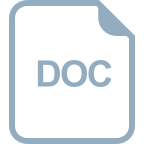
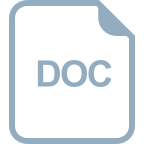
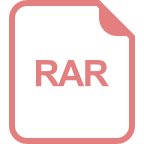
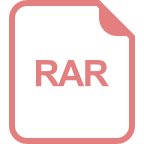







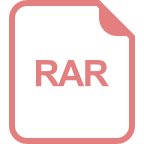
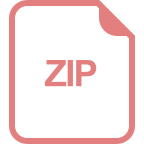
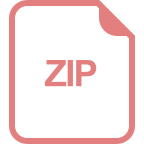
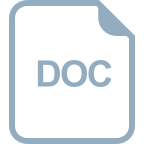
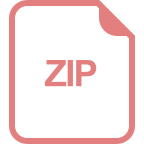
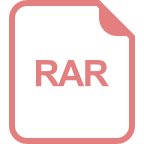
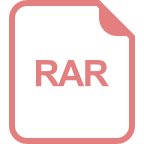