帮我搭建一个Winform程序,目的是实现串口通讯
时间: 2024-05-01 15:16:38 浏览: 11
以下是一个简单的Winform程序,实现串口通讯的功能:
1. 打开Visual Studio,选择新建项目,选择Visual C#,选择Windows桌面,选择Windows窗体应用程序,命名为SerialPortDemo。
2. 在窗体上添加以下控件:ComboBox(选择串口号)、ComboBox(选择波特率)、Button(打开串口)、Button(关闭串口)、TextBox(接收数据)、TextBox(发送数据)、Button(发送数据)。
3. 在窗体的Load事件中,初始化串口号和波特率的ComboBox控件。
```
private void Form1_Load(object sender, EventArgs e)
{
// 初始化串口号ComboBox控件
string[] ports = SerialPort.GetPortNames();
Array.Sort(ports);
comboBoxPort.Items.AddRange(ports);
// 初始化波特率ComboBox控件
comboBoxBaudRate.Items.Add("9600");
comboBoxBaudRate.Items.Add("19200");
comboBoxBaudRate.Items.Add("38400");
comboBoxBaudRate.Items.Add("57600");
comboBoxBaudRate.Items.Add("115200");
comboBoxBaudRate.SelectedIndex = 0;
}
```
4. 在打开串口按钮的Click事件中,打开选择的串口并设置相关参数。
```
private void buttonOpen_Click(object sender, EventArgs e)
{
try
{
// 打开串口
serialPort1.PortName = comboBoxPort.Text;
serialPort1.BaudRate = Convert.ToInt32(comboBoxBaudRate.Text);
serialPort1.Open();
// 设置按钮状态
buttonOpen.Enabled = false;
buttonClose.Enabled = true;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
5. 在关闭串口按钮的Click事件中,关闭打开的串口。
```
private void buttonClose_Click(object sender, EventArgs e)
{
// 关闭串口
serialPort1.Close();
// 设置按钮状态
buttonOpen.Enabled = true;
buttonClose.Enabled = false;
}
```
6. 在发送数据按钮的Click事件中,向串口发送数据。
```
private void buttonSend_Click(object sender, EventArgs e)
{
try
{
// 发送数据
serialPort1.Write(textBoxSend.Text);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
7. 在串口数据接收事件中,将接收到的数据显示在接收数据的TextBox控件中。
```
private void serialPort1_DataReceived(object sender, SerialDataReceivedEventArgs e)
{
try
{
// 接收数据
string data = serialPort1.ReadExisting();
// 显示数据
Invoke(new Action(() => { textBoxReceive.AppendText(data); }));
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
8. 在窗体的Closing事件中,关闭打开的串口。
```
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (serialPort1.IsOpen)
{
serialPort1.Close();
}
}
```
以上就是一个简单的Winform程序,实现串口通讯的功能。
相关推荐
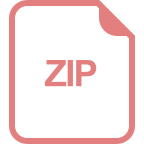
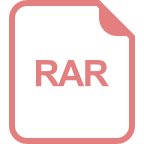














