输入一个学生的生日和当前日期,输出该学生的实足年龄
时间: 2023-05-12 18:02:44 浏览: 74
你可以使用以下代码来计算学生的实足年龄:
```python
from datetime import datetime
def calculate_age(birthdate, currentdate):
birthdate = datetime.strptime(birthdate, '%Y-%m-%d')
currentdate = datetime.strptime(currentdate, '%Y-%m-%d')
age = currentdate.year - birthdate.year - ((currentdate.month, currentdate.day) < (birthdate.month, birthdate.day))
return age
```
其中,`birthdate` 和 `currentdate` 都是字符串类型,格式为 `%Y-%m-%d`,例如 `'2000-01-01'`。你可以调用这个函数来计算学生的实足年龄,例如:
```python
birthdate = '2000-01-01'
currentdate = '2021-10-01'
age = calculate_age(birthdate, currentdate)
print(age) # 输出 21
```
注意,这个函数只能计算实足年龄,不考虑具体的出生时间。如果需要考虑出生时间,可以使用更复杂的算法。
相关问题
用python写一个代码:输入一个学生的生日和当前日期,输出该学生的实足年龄
以下是用 Python 编写的代码,可以输入学生的生日和当前日期,然后输出该学生的实足年龄:
```python
from datetime import datetime
def calculate_age(birth_date, current_date):
birth_year, birth_month, birth_day = map(int, birth_date.split('-'))
current_year, current_month, current_day = map(int, current_date.split('-'))
age = current_year - birth_year
if (current_month, current_day) < (birth_month, birth_day):
age -= 1
return age
birth_date = input("请输入学生的生日(格式为YYYY-MM-DD):")
current_date = datetime.now().strftime("%Y-%m-%d")
age = calculate_age(birth_date, current_date)
print("该学生的实足年龄为:", age, "岁")
```
请注意,这个代码假设输入的日期格式为 "YYYY-MM-DD",并且假设输入的日期是合法的。如果输入的日期格式不正确或者输入的日期不合法,那么这个代码可能会出错。
使用Python,输入一个学生的生日和当前日期,输出该学生的实际年龄
好的,我可以帮你写一个Python程序来实现这个功能。你需要提供学生的生日和当前日期,以便计算实际年龄。以下是代码示例:
```python
from datetime import date
def calculate_age(birthdate, current_date):
"""
计算一个人的实际年龄
:param birthdate: 生日,格式为 (年, 月, 日)
:param current_date: 当前日期,格式为 (年, 月, 日)
:return: 年龄
"""
birth_year, birth_month, birth_day = birthdate
current_year, current_month, current_day = current_date
age = current_year - birth_year
if (current_month, current_day) < (birth_month, birth_day):
age -= 1
return age
# 测试代码
birthdate = (2000, 1, 1)
today = date.today()
current_date = (today.year, today.month, today.day)
age = calculate_age(birthdate, current_date)
print(f"该学生的实际年龄是 {age} 岁")
```
你只需要将上面的代码粘贴到Python文件中并运行,然后输入学生的生日和当前日期即可获得该学生的实际年龄。
相关推荐
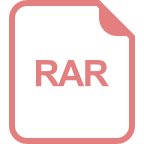












