用c++组建一个小型题库,实现组卷和判卷功能,具体要求如下: (1)建立三个文件,分别用于存放选择、判断和填空题各20道以上; (2)根据要求,从每种题型中随机抽取若干题目(可指定数量)组织试卷, 组卷后输出结果到另一文件中; (3)对于已经完成的答卷,程序自动判卷,最后给出得分。 (4)可以利用MFC进行编程,设计可视化的操作界面。
时间: 2024-02-20 21:02:20 浏览: 21
好的,这是一个比较复杂的问题,需要一步步实现。首先,我们需要准备好题库,建立三个文件,分别存放选择、判断和填空题各20道以上。假设我们把这三个文件命名为"choice.txt"、"judge.txt"和"fill-in-the-blank.txt",并将它们放在程序的同一目录下。
接着,我们需要编写程序读取这三个文件中的题目,并进行随机抽取。为了方便,我们可以将每个题目存储为一个字符串,然后将这些字符串存储在一个vector中。以下是读取文件的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
vector<string> readQuestionsFromFile(string filename) {
vector<string> questions;
ifstream fin(filename);
string line;
while (getline(fin, line)) {
questions.push_back(line);
}
fin.close();
return questions;
}
vector<string> choiceQuestions = readQuestionsFromFile("choice.txt");
vector<string> judgeQuestions = readQuestionsFromFile("judge.txt");
vector<string> fillInBlankQuestions = readQuestionsFromFile("fill-in-the-blank.txt");
int main() {
// TODO: 随机抽取题目并组卷
return 0;
}
```
接下来,我们需要随机抽取一定数量的题目,并将它们组织成试卷。我们可以用一个二维数组来存储试卷,其中第一维表示题目的类型(0代表选择题,1代表判断题,2代表填空题),第二维表示题目的编号。以下是组卷的示例代码:
```c++
const int NUM_CHOICE_QUESTIONS = 20;
const int NUM_JUDGE_QUESTIONS = 20;
const int NUM_FILL_IN_BLANK_QUESTIONS = 20;
const int NUM_QUESTIONS = NUM_CHOICE_QUESTIONS + NUM_JUDGE_QUESTIONS + NUM_FILL_IN_BLANK_QUESTIONS;
const int NUM_CHOICE_QUESTIONS_IN_EXAM = 5;
const int NUM_JUDGE_QUESTIONS_IN_EXAM = 5;
const int NUM_FILL_IN_BLANK_QUESTIONS_IN_EXAM = 5;
const int NUM_QUESTIONS_IN_EXAM = NUM_CHOICE_QUESTIONS_IN_EXAM + NUM_JUDGE_QUESTIONS_IN_EXAM + NUM_FILL_IN_BLANK_QUESTIONS_IN_EXAM;
string exam[NUM_QUESTIONS_IN_EXAM];
srand(time(NULL));
// 随机抽取选择题
for (int i = 0; i < NUM_CHOICE_QUESTIONS_IN_EXAM; i++) {
int questionIndex = rand() % NUM_CHOICE_QUESTIONS;
exam[i] = choiceQuestions[questionIndex];
}
// 随机抽取判断题
for (int i = 0; i < NUM_JUDGE_QUESTIONS_IN_EXAM; i++) {
int questionIndex = rand() % NUM_JUDGE_QUESTIONS + NUM_CHOICE_QUESTIONS;
exam[NUM_CHOICE_QUESTIONS_IN_EXAM + i] = judgeQuestions[questionIndex];
}
// 随机抽取填空题
for (int i = 0; i < NUM_FILL_IN_BLANK_QUESTIONS_IN_EXAM; i++) {
int questionIndex = rand() % NUM_FILL_IN_BLANK_QUESTIONS + NUM_CHOICE_QUESTIONS + NUM_JUDGE_QUESTIONS;
exam[NUM_CHOICE_QUESTIONS_IN_EXAM + NUM_JUDGE_QUESTIONS_IN_EXAM + i] = fillInBlankQuestions[questionIndex];
}
```
最后,我们需要实现判卷功能。由于不同类型的题目判卷方式不同,我们需要编写不同的函数来判卷。以下是判断选择题的示例代码:
```c++
int score = 0;
for (int i = 0; i < NUM_CHOICE_QUESTIONS_IN_EXAM; i++) {
string question = exam[i];
// TODO: 从题目中提取出选项和答案
// ...
cout << question << endl;
string answer;
cin >> answer;
if (answer == correctAnswer) {
score += 2;
}
}
cout << "得分:" << score << endl;
```
至此,我们已经完成了一个简单的组卷和判卷程序。如果需要使用MFC进行编程,可以考虑使用对话框来实现可视化的操作界面。
相关推荐
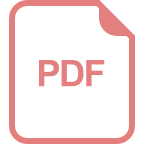
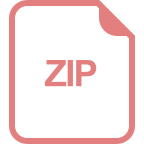
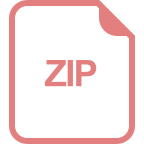














