3、自编一个小程序,读入用户输入的一个字符,按以下规则输出: 1)如果是大写字母,则输出对应的小写字母; 2)如果是小写字母,则输出对应的大写字母; 3)否则,照原字符输出。 提示:使用库函数getchar()、tolower、toupper、islower、isupper、isgraph等。
时间: 2024-03-10 20:50:00 浏览: 35
好的,下面是这个小程序的代码:
```c
#include <stdio.h>
#include <ctype.h>
int main()
{
char ch;
printf("请输入一个字符:");
ch = getchar();
if (isupper(ch)) {
ch = tolower(ch);
} else if (islower(ch)) {
ch = toupper(ch);
}
if (isgraph(ch)) {
printf("%c\n", ch);
}
return 0;
}
```
程序首先提示用户输入一个字符,然后使用 `getchar()` 函数读取用户输入的字符。接着使用 `isupper()` 和 `islower()` 函数判断字符是大写字母还是小写字母,然后使用 `toupper()` 和 `tolower()` 函数将字符转换为对应的大小写字母。最后使用 `isgraph()` 函数判断字符是否是可打印字符,如果是则输出。
相关问题
自编一个小程序,读入用户输入的一个字符,按以下规则输出: 1)如果是大写字母,则输出对应的小写字母; 2)如果是小写字母,则输出对应的大写字母; 3)否则,照原字符输出。
好的,这是一个简单的程序,可以使用 Python 语言来实现,代码如下:
```python
ch = input("请输入一个字符:")
if ch.isupper():
print(ch.lower())
elif ch.islower():
print(ch.upper())
else:
print(ch)
```
这个程序先通过 `input()` 函数读入用户输入的字符,然后通过 `isupper()` 和 `islower()` 方法判断字符的大小写,最后根据题目要求输出对应的大小写字母或原字符。
自编一个C语言小程序,读入用户输入的一个字符,按以下规则输出: 1)如果是大写字母,则输出对应的小写字母; 2)如果是小写字母,则输出对应的大写字母; 3)否则,照原字符输出。
好的,这是一个简单的程序,可以使用 C 语言来实现,代码如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char ch;
printf("请输入一个字符:");
scanf("%c", &ch);
if (isupper(ch)) {
printf("%c\n", tolower(ch));
} else if (islower(ch)) {
printf("%c\n", toupper(ch));
} else {
printf("%c\n", ch);
}
return 0;
}
```
这个程序使用了 C 语言标准库中的 `ctype.h` 头文件中的 `isupper()`、`islower()` 和 `tolower()`、`toupper()` 函数,其中 `isupper()` 和 `islower()` 函数用于判断字符的大小写,`tolower()` 和 `toupper()` 函数用于转换字符的大小写。程序先通过 `scanf()` 函数读入用户输入的字符,然后根据题目要求输出对应的大小写字母或原字符。
相关推荐
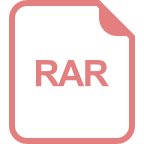
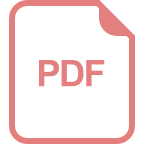












