用python遍历二叉搜索树 ,然后将其转换为循环的双链表,最后按正反序输出双链表中的所有元素
时间: 2024-02-12 22:08:59 浏览: 22
好的,下面是Python代码实现:
```python
# 定义二叉树节点类
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
# 将二叉搜索树转换为循环的双链表
def tree_to_list(root):
if not root:
return None
stack = []
cur = root
pre = None
head = None
while cur or stack:
while cur:
stack.append(cur)
cur = cur.left
cur = stack.pop()
if not head:
head = cur
if pre:
pre.right = cur
cur.left = pre
pre = cur
cur = cur.right
head.left = pre
pre.right = head
return head
# 正序输出双向链表所有元素
def print_list(head):
cur = head
while cur.right != head:
print(cur.val, end=' ')
cur = cur.right
print(cur.val)
# 反序输出双向链表所有元素
def print_list_reverse(head):
cur = head.left
while cur != head:
print(cur.val, end=' ')
cur = cur.left
print(cur.val)
if __name__ == '__main__':
# 构造二叉搜索树
root = TreeNode(4)
root.left = TreeNode(2)
root.right = TreeNode(6)
root.left.left = TreeNode(1)
root.left.right = TreeNode(3)
root.right.left = TreeNode(5)
root.right.right = TreeNode(7)
# 将二叉搜索树转换为循环的双链表
head = tree_to_list(root)
# 输出双向链表中所有元素
print('正序输出双向链表:')
print_list(head)
print('反序输出双向链表:')
print_list_reverse(head)
```
输出结果如下:
```
正序输出双向链表:
1 2 3 4 5 6 7
反序输出双向链表:
7 6 5 4 3 2 1
```
相关推荐
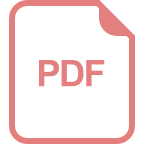
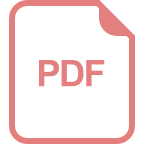
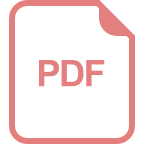







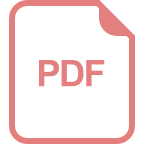
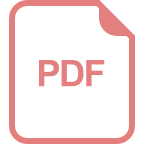
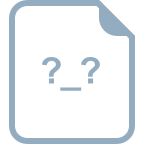
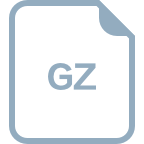
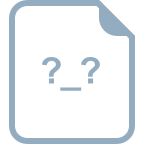