vue动态添加class类名
时间: 2023-08-27 22:22:42 浏览: 161
在Vue中动态添加class类名可以通过以下方式实现:
1. 使用对象语法:
你可以使用一个对象来指定需要添加的class类名,对象的key为类名,value为一个布尔值,如果该值为真,则添加对应的类名,如果为假,则不添加对应的类名。
```html
<template>
<div :class="{ 'active': isActive, 'highlight': isHighlighted }"></div>
</template>
<script>
export default {
data() {
return {
isActive: true,
isHighlighted: false
};
}
};
</script>
<style>
.active {
color: red;
}
.highlight {
background-color: yellow;
}
</style>
```
在上面的例子中,当isActive为true时,div元素会添加active类名,从而应用red颜色样式;当isHighlighted为false时,div元素不会添加highlight类名,从而不应用yellow背景色样式。
2. 使用数组语法:
你可以使用一个数组来指定需要添加的class类名,数组中的每个元素都可以是一个字符串或一个动态绑定的数据。
```html
<template>
<div :class="[activeClass, errorClass]"></div>
</template>
<script>
export default {
data() {
return {
activeClass: 'active',
errorClass: 'error'
};
}
};
</script>
<style>
.active {
color: red;
}
.error {
border: 1px solid red;
}
</style>
```
在上面的例子中,div元素会同时添加active和error类名,从而应用red颜色样式和红色边框样式。
3. 使用计算属性:
你可以利用计算属性来动态计算需要添加的class类名。
```html
<template>
<div :class="computedClass"></div>
</template>
<script>
export default {
data() {
return {
isActive: true,
isHighlighted: false
};
},
computed: {
computedClass() {
return {
'active': this.isActive,
'highlight': this.isHighlighted
};
}
}
};
</script>
<style>
.active {
color: red;
}
.highlight {
background-color: yellow;
}
</style>
```
在上面的例子中,计算属性computedClass会根据isActive和isHighlighted的值动态返回一个对象,然后该对象会被应用到div元素的class属性上。
以上是在Vue中动态添加class类名的几种常见方式,你可以根据具体场景选择合适的方式来实现。
阅读全文
相关推荐
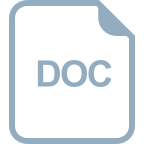
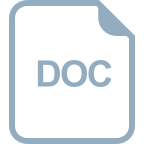
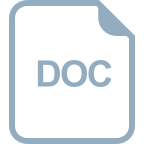

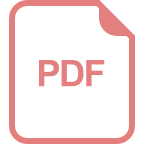













