electron 获取某个文件并上传
时间: 2024-12-15 22:31:06 浏览: 4
在 Electron 中,你可以通过 Node.js 的文件系统模块(`fs`)获取本地文件,并结合 `axios` 或其他 HTTP 库来发送文件到服务器。以下是一个基本步骤:
1. **安装所需的依赖**:首先,确保已经安装了 `fs` 和一个用于处理HTTP请求的库,如 `axios`。可以使用 npm 安装:
```bash
npm install fs axios
```
2. **读取文件**:
使用 `fs` 的 `readFile` 方法读取你需要的文件内容:
```javascript
const fs = require('fs');
const filePath = 'path/to/your/file.txt';
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) throw err;
// data 现在包含了文件的内容
});
```
3. **构造文件数据**:
将文件内容转换为适合上传的形式,比如 base64 编码、Blob 或 File 对象。
4. **发送请求**:
使用 `axios` 发送 POST 请求并将文件作为请求体:
```javascript
const axios = require('axios');
const formData = new FormData();
formData.append('file', data); // 或者 formData.append('file', Buffer.from(data), 'filename.txt'); // 如果是二进制
axios.post('https://example.com/upload', formData)
.then(response => {
console.log('Upload successful:', response.data);
})
.catch(error => {
console.error('Error uploading:', error);
});
```
请注意替换 `'https://example.com/upload'` 为你实际的目标上传地址。
阅读全文
相关推荐
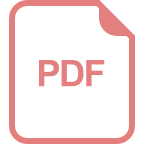
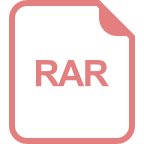
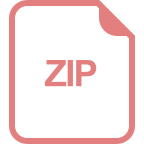

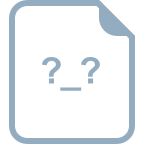
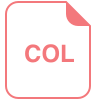
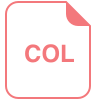
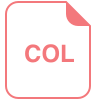
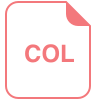
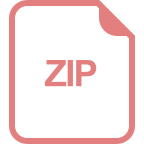
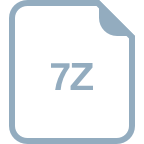
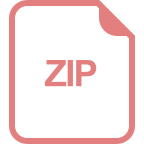