RuntimeError: result type Float can't be cast to the desired output type __int64
时间: 2023-09-23 11:07:08 浏览: 53
This error occurs when you try to convert a floating-point number to an integer value using the __int64 data type. The __int64 data type is used for 64-bit integer values, while float data type is used for decimal values.
To fix this error, you should convert the floating-point number to an integer value using the int() function instead of the __int64 data type. For example:
```python
x = 3.14
y = int(x)
print(y)
```
In this example, the float value 3.14 is converted to an integer value using the int() function, and the output will be 3.
相关问题
yolov3 RuntimeError: result type Float can't be cast to the desired output type __int64
在yolov3中,出现"RuntimeError: result type Float can't be cast to the desired output type __int64"的错误通常是由于数据类型不匹配导致的。这个错误提示指出无法将浮点数类型(Float)转换为所需的输出类型(__int64)[^1][^2]。
解决这个问题的方法是将浮点数类型转换为整数类型。你可以使用Python的内置函数int()来实现这个转换。具体的做法是在需要转换的浮点数变量前面加上int()函数,将其转换为整数类型。例如,如果你的代码中有类似于indices.append((b, a, gj.clamp(0, int(gain) - 1), gi.clamp(0, int(gain) - 1)))这样的语句,你可以将其中的浮点数变量使用int()函数进行转换,如下所示:
```python
indices.append((b, a, gj.clamp(0, int(gain) - 1), gi.clamp(0, int(gain) - 1)))
```
这样就可以将浮点数类型转换为整数类型,避免了"RuntimeError: result type Float can't be cast to the desired output type __int64"的错误。
YOLOv7训练报错RuntimeError: result type Float can't be cast to the desired output type __int64
根据提供的引用内容,你遇到的问题是YOLOv7训练报错RuntimeError: result type Float can't be cast to the desired output type __int64。这个错误是由于你的torch版本不支持将float类型直接转换为long int类型的数据。解决这个问题的方法有两种:
方法一:修改代码
在lib软件包中找到core软件包下的postprocess.py文件,找到第30行的代码:
```python
gain = torch.ones(7, device=targets.device)
```
将这行代码修改为:
```python
gain = torch.ones(7, device=targets.device).long()
```
然后重新运行train.py,这样就可以成功解决这个问题。
方法二:降低torch版本
另一种解决方法是降低torch的版本。你可以尝试使用较旧的torch版本,因为较新的torch版本可能会出现这个问题。你可以通过以下命令降低torch版本:
```shell
pip install torch==<version>
```
其中,<version>是你想要安装的torch版本号。安装完成后,重新运行train.py,应该就能够解决这个问题。
相关推荐
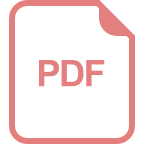
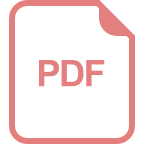
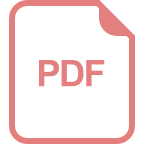












