ValueError: non-broadcastable output operand with shape (110,1) doesn't match the broadcast shape (110,4)
时间: 2024-01-24 10:04:11 浏览: 77
这个错误通常是由于数组形状不兼容而导致的。在您的代码中,似乎您正在尝试将形状为(110,1)的数组与形状为(110,4)的数组进行广播操作,从而导致此错误。
您可以尝试使用 `reshape()` 方法来重新调整数组的形状,以确保它们具有兼容的形状。例如,如果您想将形状为(110,1)的数组转换为形状为(110,4)的数组,可以使用以下代码:
```python
train_predict = np.tile(train_predict, (1, 4))
```
这将使用 `tile()` 函数将 `train_predict` 数组沿列方向复制 4 次,以便它的形状变为(110,4)。然后,您可以再次尝试执行广播操作。
相关问题
ValueError: non-broadcastable output operand with shape (1640,1) doesn't match the broadcast shape (1640,4)
This error occurs when you are trying to perform a mathematical operation that cannot be broadcasted to the output shape. In this case, the output operand has shape (1640,1) but the broadcast shape is (1640,4).
To fix this error, you can reshape the output operand to match the broadcast shape using the numpy.reshape() function. For example:
```python
import numpy as np
# create a matrix with shape (1640, 4)
a = np.ones((1640, 4))
# create an output operand with shape (1640, 1)
b = np.ones((1640, 1))
# reshape the output operand to match the broadcast shape
b = b.reshape(1640, 4)
# perform the mathematical operation
c = a + b
```
In this example, we reshape the output operand `b` to have shape (1640, 4) using the `reshape()` function. Now, we can perform the mathematical operation without getting the ValueError.
ValueError: non-broadcastable output operand with shape (45,1) doesn't match the broadcast shape (45,5)
This error occurs when you are trying to perform an operation that requires two arrays to have the same shape, but they do not. Specifically, the error message is telling you that you are trying to assign the result of an operation to an array with shape (45,1), but the operation result has shape (45,5), which cannot be broadcast to match the shape of the output array.
To fix this error, you need to make sure that the arrays you are working with have compatible shapes. One possible solution is to reshape the output array to match the shape of the operation result. For example:
```
import numpy as np
a = np.random.rand(45, 5)
b = np.random.rand(45, 1)
c = a * b.reshape(45, 1)
```
In this example, we reshape the array `b` to have shape (45, 1) so that it can be broadcast with `a`. The result of the multiplication is an array with shape (45, 5), but we can reshape it to have shape (45, 1) by taking the sum of each row:
```
d = c.sum(axis=1, keepdims=True)
```
Now `d` has shape (45, 1) and can be assigned to an array with the same shape.
相关推荐
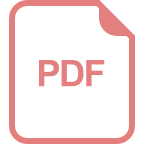
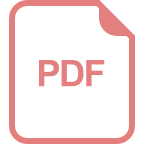
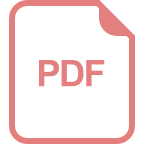













