ValueError: non-broadcastable output operand with shape (60,1) doesn't match the broadcast shape (60,5)
时间: 2023-06-15 11:06:28 浏览: 233
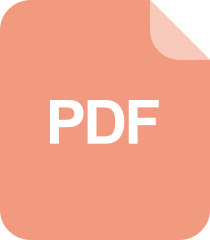
python3.7解决最小二乘遇到ValueError:Expected 2D array, got 1D array instead: array=[5.].关于reshape和predict
这个错误通常是由于在进行数组操作时,两个数组的形状不兼容导致的。更具体地讲,你试图将一个形状为(60,1)的数组广播到一个形状为(60,5)的数组上,但两个形状不匹配。这可能是因为你的代码中存在某些矩阵乘法、加法或其他数组操作,导致了形状不兼容的问题。
为了解决这个问题,你需要仔细检查你的代码,找出哪些数组操作导致了形状不兼容的问题。你可以使用 NumPy 中的 reshape() 函数来改变数组的形状,以使其与另一个数组兼容。或者你可以使用广播规则来将两个形状不同的数组进行运算。
例如,如果你有一个形状为(60,1)的数组a和一个形状为(60,5)的数组b,你可以使用广播规则将a广播到形状(60,5),以使它们兼容:
```
a = np.random.rand(60, 1)
b = np.random.rand(60, 5)
c = a * b
```
在这个例子中,a被广播到形状(60,5),然后与b相乘,得到一个形状为(60,5)的数组c。如果你仍然遇到问题,请提供更多的代码和上下文,以便我更好地帮助你解决这个问题。
阅读全文
相关推荐
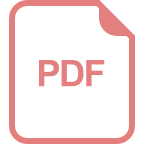
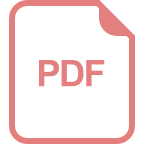
















