ValueError: non-broadcastable output operand with shape (110,1) doesn't match the broadcast shape (110,4)
时间: 2024-05-18 07:12:00 浏览: 177
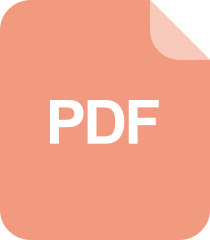
python3.7解决最小二乘遇到ValueError:Expected 2D array, got 1D array instead: array=[5.].关于reshape和predict
这个错误通常会在使用numpy的广播机制时出现。它表示输出操作数的形状不可广播到给定的形状。
例如,如果你在一个形状为(110,1)的数组上执行乘法,它将无法广播到形状为(110,4)的数组。这是因为这两个形状无法对齐。
解决办法通常是将两个数组形状对齐,或者重构数组以使其可以广播。你可以使用numpy的reshape函数或者transpose函数来实现这一点。
如果你需要更具体的解决方案,请提供更多的上下文和代码。
阅读全文
相关推荐
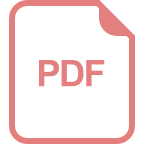
















