python调用GPU
时间: 2023-09-06 16:14:21 浏览: 167
要在Python中使用GPU,需要使用一个支持GPU加速的深度学习框架,例如TensorFlow、PyTorch等。这些框架会自动检测并利用可用的GPU来加速计算。
以下是使用TensorFlow在Python中调用GPU的示例代码:
```python
import tensorflow as tf
# 检查是否有可用的GPU
if tf.test.gpu_device_name():
print('Default GPU Device: {}'.format(tf.test.gpu_device_name()))
else:
print("Please install GPU version of TF")
# 创建一个TensorFlow会话
with tf.Session() as sess:
# 构建一个简单的计算图
a = tf.constant(10)
b = tf.constant(20)
c = tf.add(a, b)
# 运行计算图并打印结果
print(sess.run(c))
```
该代码首先检查是否有可用的GPU,然后创建一个TensorFlow会话并构建一个简单的计算图。在运行计算图时,TensorFlow会自动检测并利用可用的GPU来加速计算。
相关问题
Python 调用GPU
在Python中使用GPU进行计算通常涉及到使用特定的库,如NumPy、Pandas(它们默认是CPU加速的),或是专为GPU编程设计的库如TensorFlow、PyTorch等。如果你想要利用GPU,你需要确保你的系统已经安装了支持GPU的版本,并且正确配置了环境。
首先,你需要安装CUDA和cuDNN(对NVIDIA GPU的支持库):
```bash
# 对于NVIDIA GPU
sudo apt-get install nvidia-cuda-toolkit # Ubuntu
# 或者 yum install nvidia-cuda # CentOS/RHEL
```
然后安装Python GPU库,例如:
- TensorFlow: `pip install tensorflow-gpu`
- PyTorch: `pip install torch torchvision torchaudio -f https://download.pytorch.org/whl/cpu/torch_stable.html`
为了使用GPU,你需要在代码中明确指定:
```python
import tensorflow as tf
physical_devices = tf.config.list_physical_devices('GPU')
if physical_devices:
tf.config.experimental.set_memory_growth(physical_devices, True)
print("Using GPU:", tf.test.is_gpu_available())
# 或者 PyTorch
import torch
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print("Using GPU:", device.type == 'cuda')
```
python调用gpu
要使用GPU进行Python编程,你需要安装适当的GPU驱动程序和CUDA工具包。以下是一些步骤:
1. 确保你的计算机上有一块支持CUDA的NVIDIA GPU。你可以在NVIDIA官网上查找你的GPU型号是否支持CUDA。
2. 安装适当的NVIDIA GPU驱动程序。你可以从NVIDIA官网下载并安装最新的GPU驱动程序。
3. 安装CUDA工具包。CUDA是NVIDIA提供的用于并行计算的开发平台和API。你可以从NVIDIA官网下载并安装适合你的GPU型号的CUDA工具包版本。
4. 安装GPU支持的Python库。一些常见的GPU支持的Python库包括TensorFlow、PyTorch和MXNet。你可以使用pip命令来安装它们,例如:
```
pip install tensorflow-gpu
pip install torch torchvision
pip install mxnet-cuXXX # 这里的XXX是你的CUDA版本号,例如mxnet-cu111
```
5. 配置环境变量。将CUDA和GPU支持的Python库的路径添加到系统的环境变量中,以便Python能够找到它们。
完成上述步骤后,你就可以在Python中使用GPU进行加速计算了。请注意,具体的使用方法和代码可能因不同的库和任务而有所不同,你可以参考相应库的文档和示例来学习如何使用GPU进行计算。
阅读全文
相关推荐
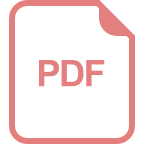
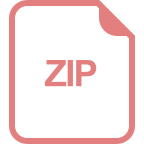




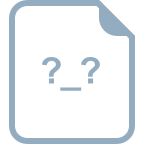








