如何在surfaceMeanSize中调用Cone方法中的radius?public class Cone extends GraphicObject{ public Cone(ObjectColor color, double radius, double length) { } @Override public double surfaceMeanSize() { return Math.PI * () } @Override public double volume() { return 0; }
时间: 2023-06-24 13:08:59 浏览: 34
您可以将Cone类中的radius作为属性保存,并将其传递给surfaceMeanSize()方法。在这种情况下,您可以使用以下代码:
public class Cone extends GraphicObject{
private double radius;
private double length;
public Cone(ObjectColor color, double radius, double length) {
this.radius = radius;
this.length = length;
}
@Override
public double surfaceMeanSize() {
double slantHeight = Math.sqrt(Math.pow(radius, 2) + Math.pow(length, 2));
return Math.PI * radius * slantHeight;
}
@Override
public double volume() {
return 0;
}
}
在这个例子中,我们使用了Cone类的构造函数来设置radius和length属性,并在surfaceMeanSize()方法中使用它们计算圆锥的表面积。
相关问题
complete abstract class GraphicObject and implement subclasses Cone , Cuboid and Sphere based on the class GraphicObject .
Here is an example implementation of the abstract class GraphicObject and its subclasses Cone, Cuboid, and Sphere:
```java
abstract class GraphicObject {
protected int x, y;
public GraphicObject(int x, int y) {
this.x = x;
this.y = y;
}
abstract double area();
abstract double perimeter();
}
class Cone extends GraphicObject {
private int radius;
private int height;
public Cone(int x, int y, int radius, int height) {
super(x, y);
this.radius = radius;
this.height = height;
}
@Override
double area() {
return Math.PI * radius * (radius + Math.sqrt(Math.pow(height, 2) + Math.pow(radius, 2)));
}
@Override
double perimeter() {
return 2 * Math.PI * radius;
}
}
class Cuboid extends GraphicObject {
private int length;
private int width;
private int height;
public Cuboid(int x, int y, int length, int width, int height) {
super(x, y);
this.length = length;
this.width = width;
this.height = height;
}
@Override
double area() {
return 2 * (length * width + width * height + height * length);
}
@Override
double perimeter() {
return 4 * (length + width + height);
}
}
class Sphere extends GraphicObject {
private int radius;
public Sphere(int x, int y, int radius) {
super(x, y);
this.radius = radius;
}
@Override
double area() {
return 4 * Math.PI * Math.pow(radius, 2);
}
@Override
double perimeter() {
return 2 * Math.PI * radius;
}
}
```
The abstract class `GraphicObject` defines the common properties and methods that all subclasses must implement. The `Cone`, `Cuboid`, and `Sphere` classes extend the `GraphicObject` class and provide their own implementations of the `area()` and `perimeter()` methods.
For example, the `Cone` class calculates the surface area and circumference of a cone based on its radius and height, while the `Cuboid` class calculates the surface area and perimeter of a rectangular cuboid based on its length, width, and height. The `Sphere` class calculates the surface area and circumference of a sphere based on its radius.
Note that the `GraphicObject` class is abstract and cannot be instantiated directly. Instead, it provides a template for creating subclasses that represent specific types of geometric objects.
1.写一个锥体的泛型类Cone<E>,泛型E为它的底面,Cone可以设置高度和计算体积。然后定义一个泛型Circle。在主类中让Circle作为Cone<E>的底面,并计算Cone<E>的体积。使用java编写
好的,下面是代码实现:
定义泛型类Circle:
```java
public class Circle<T extends Number> {
private T radius;
public Circle(T radius) {
this.radius = radius;
}
public T getRadius() {
return radius;
}
public void setRadius(T radius) {
this.radius = radius;
}
}
```
定义泛型类Cone:
```java
public class Cone<E extends Circle<? extends Number>> {
private E bottom;
private Number height;
public Cone(E bottom, Number height) {
this.bottom = bottom;
this.height = height;
}
public E getBottom() {
return bottom;
}
public void setBottom(E bottom) {
this.bottom = bottom;
}
public Number getHeight() {
return height;
}
public void setHeight(Number height) {
this.height = height;
}
public double getVolume() {
double r = bottom.getRadius().doubleValue();
double h = height.doubleValue();
return 1.0 / 3.0 * Math.PI * r * r * h;
}
}
```
在主函数中使用Circle作为Cone的底面并计算体积:
```java
public class Main {
public static void main(String[] args) {
Circle<Double> circle = new Circle<>(2.0);
Cone<Circle<Double>> cone = new Cone<>(circle, 4.0);
System.out.println("Cone Volume: " + cone.getVolume());
}
}
```
输出结果:Cone Volume: 16.755160819145562
注意:这里的泛型类Cone的底面必须是Circle的子类,而Circle的泛型参数必须是Number的子类,因为在计算体积时需要进行类型转换。
相关推荐
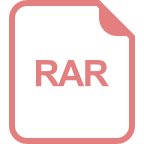
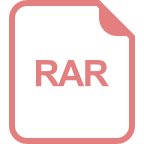









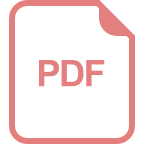
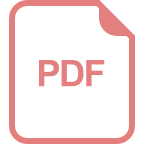