使用xml方式实现的简单Spring AOP实例
时间: 2023-08-23 16:05:53 浏览: 51
以下是一个使用 XML 方式实现的简单 Spring AOP 示例:
首先,需要定义一个切面类和一个目标类。假设切面类是 LoggingAspect,目标类是 HelloWorld。
LoggingAspect.java:
```java
public class LoggingAspect {
public void beforeAdvice() {
System.out.println("Going to setup student profile.");
}
public void afterAdvice() {
System.out.println("Student profile has been setup.");
}
}
```
HelloWorld.java:
```java
public class HelloWorld {
private String message;
public void setMessage(String message) {
this.message = message;
}
public void getMessage() {
System.out.println("Your Message : " + message);
}
}
```
然后,在 Spring 配置文件中定义切面和目标对象,并将它们织入到一起。在此示例中,我们使用 XML 配置 AOP。
applicationContext.xml:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd">
<bean id="helloWorld" class="com.example.HelloWorld">
<property name="message" value="Hello World!" />
</bean>
<bean id="loggingAspect" class="com.example.LoggingAspect" />
<aop:config>
<aop:aspect id="aspect" ref="loggingAspect">
<aop:pointcut id="allMethods"
expression="execution(* com.example.HelloWorld.*(..))" />
<aop:before pointcut-ref="allMethods" method="beforeAdvice" />
<aop:after pointcut-ref="allMethods" method="afterAdvice" />
</aop:aspect>
</aop:config>
</beans>
```
在此配置文件中,我们定义了一个名为 helloWorld 的 bean(即目标对象)和一个名为 loggingAspect 的 bean(即切面)。然后,我们使用 aop:config 元素定义了一个切面,其中包含一个 pointcut(即 allMethods),它定义了要拦截的方法。我们还使用 aop:before 和 aop:after 元素定义了要在目标方法之前和之后执行的通知(即 beforeAdvice 和 afterAdvice 方法)。
最后,在应用程序中加载 Spring 配置文件,并使用它来获取 HelloWorld bean 并调用 getMessage 方法:
```java
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class App {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
HelloWorld obj = (HelloWorld) context.getBean("helloWorld");
obj.getMessage();
}
}
```
输出将如下所示:
```
Going to setup student profile.
Your Message : Hello World!
Student profile has been setup.
```
相关推荐
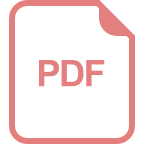
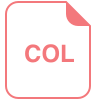
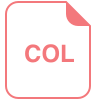
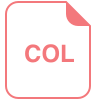
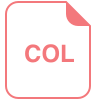
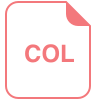









