Spring AOP完整例子
时间: 2023-10-26 12:13:52 浏览: 115
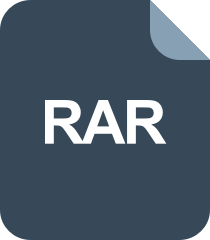
Spring AOP实例
我们来实现一个简单的 Spring AOP 的例子,假设我们有一个计算器类 Calculator,它有两个方法 add 和 subtract,我们想要在这两个方法执行前后打印日志。
首先,我们需要定义一个切面类 LogAspect,它包含两个方法,一个执行前的方法 before,一个执行后的方法 after。
```java
@Aspect
@Component
public class LogAspect {
@Before("execution(* com.example.demo.Calculator.*(..))")
public void before(JoinPoint joinPoint) {
System.out.println("before " + joinPoint.getSignature().getName());
}
@After("execution(* com.example.demo.Calculator.*(..))")
public void after(JoinPoint joinPoint) {
System.out.println("after " + joinPoint.getSignature().getName());
}
}
```
我们使用 @Aspect 注解将这个类标记为切面类,使用 @Before 和 @After 注解分别标记 before 和 after 方法,它们的参数是一个切入点表达式,表示在哪些方法执行前或执行后应该执行这个方法。这里我们使用了 execution(* com.example.demo.Calculator.*(..)) 表达式,它表示在 Calculator 类中的所有方法执行前或执行后都要执行这个方法。
接下来,我们需要在 Spring 的配置文件中声明这个切面类,并开启 AOP 功能。假设我们的配置文件名为 application-context.xml,内容如下:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.2.xsd">
<bean id="calculator" class="com.example.demo.Calculator"/>
<bean id="logAspect" class="com.example.demo.LogAspect"/>
<aop:aspectj-autoproxy/>
</beans>
```
我们使用 <bean> 标签声明一个 Calculator 类的实例,并使用 <bean> 标签声明一个 LogAspect 类的实例。然后使用 <aop:aspectj-autoproxy> 标签开启 AOP 功能。这里需要注意,我们使用了 AspectJ 注解来声明切面类和切入点表达式,所以需要使用 <aop:aspectj-autoproxy> 标签来开启 AspectJ 支持。
最后,我们在程序中使用 Spring 的实例工厂来获取 Calculator 类的实例,并使用它的 add 和 subtract 方法进行测试。
```java
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("application-context.xml");
Calculator calculator = (Calculator) context.getBean("calculator");
calculator.add(1, 2);
calculator.subtract(2, 1);
}
}
```
运行程序,我们应该可以看到如下的输出:
```
before add
after add
before subtract
after subtract
```
这表示我们的切面类 LogAspect 成功地拦截了 Calculator 类中的 add 和 subtract 方法的执行,并在它们执行前后打印了日志。
阅读全文
相关推荐
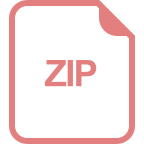
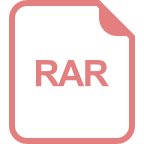
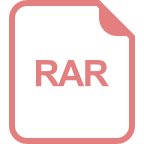
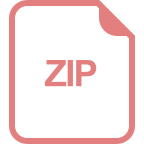
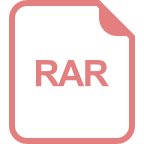
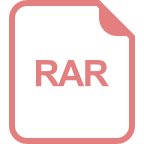
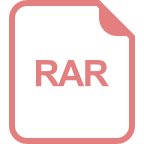
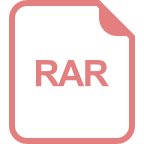
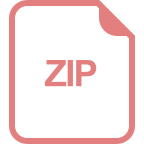
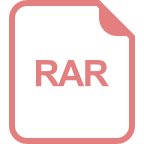
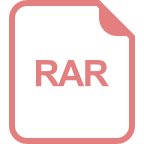
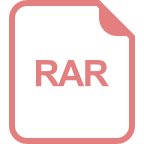
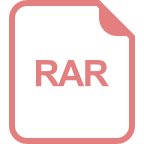
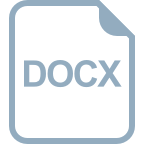
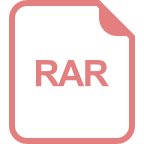