spring boot aop 教程
时间: 2023-10-20 11:08:28 浏览: 67
Spring Boot AOP(面向切面编程)是一种在Spring Boot应用中实现横切关注点的技术。它允许开发者在应用的多个地方插入额外的逻辑,而不需要修改原始代码。下面是一个简单的Spring Boot AOP教程:
1. 添加依赖:在你的Spring Boot项目的pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
```
2. 创建切面类:创建一个Java类,并使用`@Aspect`注解标记它作为一个切面类。在切面类中,你可以定义各种通知(advice)和切点(pointcut)。例如:
```java
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().getName());
}
@AfterReturning(pointcut = "execution(* com.example.service.*.*(..))", returning = "result")
public void logAfterReturning(JoinPoint joinPoint, Object result) {
System.out.println("After returning method: " + joinPoint.getSignature().getName());
System.out.println("Result: " + result);
}
// 其他通知和切点的定义...
}
```
在上面的例子中,`logBefore()`方法是一个在目标方法执行之前执行的前置通知(Before Advice),`logAfterReturning()`方法是一个在目标方法执行之后执行的后置通知(After Returning Advice)。
3. 启用AOP:在Spring Boot应用的主类上添加`@EnableAspectJAutoProxy`注解,以启用AOP:
```java
@SpringBootApplication
@EnableAspectJAutoProxy
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
```
4. 测试AOP:运行应用,并调用被切入的方法,你将看到相关的日志输出。
这只是一个简单的Spring Boot AOP教程,AOP还有很多其他的功能和概念,例如切面优先级、环绕通知等等。你可以进一步学习和探索Spring Boot AOP的更多功能和用法。希望对你有所帮助!
相关推荐
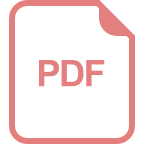













