写一篇基于python的象棋游戏的代码
时间: 2024-03-14 14:44:58 浏览: 14
抱歉,由于时间和空间的限制,我无法在此处为您提供完整的Python象棋游戏代码。但是,我可以为您提供一个基于Python的象棋游戏代码框架,您可以在此基础上进行修改和完善。
首先,我们需要定义棋盘和棋子。我们可以使用列表来表示棋盘,每个元素代表一个棋子的位置。用字母表示棋子:
```python
chess_board = [
['車', '馬', '象', '士', '将', '士', '象', '馬', '車'],
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '],
[' ', '炮', ' ', ' ', ' ', ' ', ' ', '炮', ' '],
['卒', ' ', '卒', ' ', '卒', ' ', '卒', ' ', '卒'],
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '],
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '],
['兵', ' ', '兵', ' ', '兵', ' ', '兵', ' ', '兵'],
[' ', '砲', ' ', ' ', ' ', ' ', ' ', '砲', ' '],
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '],
['俥', '傌', '相', '仕', '帥', '仕', '相', '傌', '俥'],
]
```
使用一个字典来表示每个棋子的类型和颜色:
```python
piece = {
'車': {'color': 'red', 'type': 'car'},
'馬': {'color': 'red', 'type': 'horse'},
'象': {'color': 'red', 'type': 'elephant'},
'士': {'color': 'red', 'type': 'guard'},
'将': {'color': 'red', 'type': 'general'},
'炮': {'color': 'red', 'type': 'cannon'},
'卒': {'color': 'red', 'type': 'pawn'},
'俥': {'color': 'black', 'type': 'car'},
'傌': {'color': 'black', 'type': 'horse'},
'相': {'color': 'black', 'type': 'elephant'},
'仕': {'color': 'black', 'type': 'guard'},
'帥': {'color': 'black', 'type': 'general'},
'砲': {'color': 'black', 'type': 'cannon'},
'兵': {'color': 'black', 'type': 'pawn'},
}
```
接下来,我们需要实现棋子的移动。我们可以使用一个函数来检查棋子是否能够移动到目标位置:
```python
def can_move(piece, target_x, target_y):
if target_x < 0 or target_x > 8 or target_y < 0 or target_y > 9:
return False
if piece['type'] == 'car':
if target_x != piece['x'] and target_y != piece['y']:
return False
if target_x == piece['x']:
step = 1 if target_y > piece['y'] else -1
for i in range(piece['y'] + step, target_y, step):
if chess_board[piece['x']][i] != ' ':
return False
else:
step = 1 if target_x > piece['x'] else -1
for i in range(piece['x'] + step, target_x, step):
if chess_board[i][piece['y']] != ' ':
return False
return True
elif piece['type'] == 'horse':
dx = target_x - piece['x']
dy = target_y - piece['y']
if dx == 2 and dy == 1 and chess_board[piece['x'] + 1][piece['y']] == ' ':
return True
if dx == 2 and dy == -1 and chess_board[piece['x'] + 1][piece['y']] == ' ':
return True
if dx == -2 and dy == 1 and chess_board[piece['x'] - 1][piece['y']] == ' ':
return True
if dx == -2 and dy == -1 and chess_board[piece['x'] - 1][piece['y']] == ' ':
return True
if dx == 1 and dy == 2 and chess_board[piece['x']][piece['y'] + 1] == ' ':
return True
if dx == 1 and dy == -2 and chess_board[piece['x']][piece['y'] - 1] == ' ':
return True
if dx == -1 and dy == 2 and chess_board[piece['x']][piece['y'] + 1] == ' ':
return True
if dx == -1 and dy == -2 and chess_board[piece['x']][piece['y'] - 1] == ' ':
return True
return False
# 其他棋子的移动规则
```
最后,我们需要实现游戏的交互和逻辑。我们可以使用一个循环来实现不断的游戏交互:
```python
def play_game():
turn = 'red'
while True:
draw_board()
input_x = input('请输入要移动的棋子的x坐标(1-9):')
input_y = input('请输入要移动的棋子的y坐标(1-10):')
target_x = input('请输入目标位置的x坐标(1-9):')
target_y = input('请输入目标位置的y坐标(1-10):')
piece = chess_board[input_x - 1][input_y - 1]
if piece == ' ':
print('该位置没有棋子,请重新输入')
continue
if piece['color'] != turn:
print('该棋子不是你的,请重新输入')
continue
if not can_move(piece, target_x - 1, target_y - 1):
print('该棋子不能移动到目标位置,请重新输入')
continue
chess_board[target_x - 1][target_y - 1] = piece
chess_board[input_x - 1][input_y - 1] = ' '
turn = 'red' if turn == 'black' else 'black'
```
这是一个简单的Python象棋游戏框架,您可以在此基础上进行修改和完善。
相关推荐
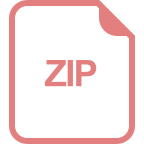
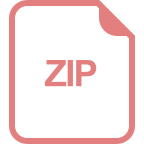















