用java语言写。For this task you are required to write a class to represent a Dice. Your class should contain all features and functionality of a Die. Once you have your Dice class written you are going to write a very simple game. The game is one where each player has their own n sided Dice, and they win the game by being the first to reach a cumulative score after rolling their Dice. Your game should allow Dice the same n-sided dice for each player. The game should allow the number of players and the winning score to be decided by the user. It should print out the winning player the score they achieved and how many rolls it took them to achieve their score.
时间: 2024-02-14 20:02:50 浏览: 20
Sure, I can help you with that. Here is a basic implementation of the Dice class in Java:
```java
import java.util.Random;
public class Dice {
private int sides;
private Random rand;
public Dice(int sides) {
this.sides = sides;
this.rand = new Random();
}
public int roll() {
return rand.nextInt(sides) + 1;
}
}
```
This class has a constructor that takes the number of sides on the die, and a `roll()` method that returns a random number between 1 and the number of sides.
Now, let's write the game that uses this class:
```java
import java.util.Scanner;
public class DiceGame {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the number of players: ");
int numPlayers = scanner.nextInt();
System.out.print("Enter the number of sides on each die: ");
int numSides = scanner.nextInt();
System.out.print("Enter the winning score: ");
int winningScore = scanner.nextInt();
Dice[] dice = new Dice[numPlayers];
int[] scores = new int[numPlayers];
for (int i = 0; i < numPlayers; i++) {
dice[i] = new Dice(numSides);
}
int winner = -1;
int rolls = 0;
while (winner == -1) {
for (int i = 0; i < numPlayers; i++) {
int roll = dice[i].roll();
scores[i] += roll;
rolls++;
if (scores[i] >= winningScore) {
winner = i;
break;
}
}
}
System.out.println("Player " + (winner + 1) + " wins with a score of " + scores[winner] + " in " + rolls + " rolls.");
}
}
```
This game prompts the user for the number of players, the number of sides on each die, and the winning score. It then creates an array of `Dice` objects and an array to store each player's score. It then enters a loop that rolls the dice for each player and updates their scores until a player reaches the winning score. Once a winner is found, the game prints out the winner's information.
I hope that helps! Let me know if you have any questions.
相关推荐
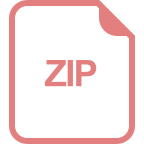
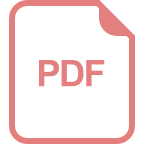
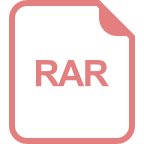














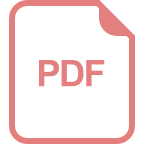