你可以用python+tkinter写一个图书借阅管理系统吗
时间: 2024-05-06 13:15:05 浏览: 121
当然可以,Python 是一门功能强大的编程语言,也有许多图形界面库可以用来编写应用程序,其中 Tkinter 是 Python 自带的 GUI 库,非常容易上手。下面是一个简单的图书借阅管理系统的例子,仅供参考:
```python
from tkinter import *
import sqlite3
# 创建数据库连接
conn = sqlite3.connect('library.db')
c = conn.cursor()
# 创建图书表
c.execute('''CREATE TABLE IF NOT EXISTS books
(id INTEGER PRIMARY KEY,
title TEXT,
author TEXT,
year INTEGER,
status TEXT)''')
# 创建借阅表
c.execute('''CREATE TABLE IF NOT EXISTS loans
(id INTEGER PRIMARY KEY,
book_id INTEGER,
borrower TEXT,
borrow_date TEXT,
return_date TEXT)''')
# 初始化窗口
root = Tk()
root.title("图书借阅管理系统")
root.geometry("500x400")
# 标签
title_label = Label(root, text="图书借阅管理系统", font=("Arial", 20))
title_label.pack(pady=10)
# 输入框和标签
title_var = StringVar()
title_label = Label(root, text="书名:")
title_label.pack(pady=5)
title_entry = Entry(root, textvariable=title_var)
title_entry.pack()
author_var = StringVar()
author_label = Label(root, text="作者:")
author_label.pack(pady=5)
author_entry = Entry(root, textvariable=author_var)
author_entry.pack()
year_var = IntVar()
year_label = Label(root, text="年份:")
year_label.pack(pady=5)
year_entry = Entry(root, textvariable=year_var)
year_entry.pack()
# 添加图书按钮
def add_book():
title = title_var.get()
author = author_var.get()
year = year_var.get()
status = "在库"
c.execute("INSERT INTO books (title, author, year, status) VALUES (?, ?, ?, ?)",
(title, author, year, status))
conn.commit()
message_label.config(text="添加图书成功")
add_button = Button(root, text="添加图书", command=add_book)
add_button.pack(pady=10)
# 消息标签
message_label = Label(root, text="")
message_label.pack(pady=5)
# 查询图书按钮
def search_books():
title = title_var.get()
author = author_var.get()
year = year_var.get()
where_clause = []
if title:
where_clause.append("title LIKE '%{}%'".format(title))
if author:
where_clause.append("author LIKE '%{}%'".format(author))
if year:
where_clause.append("year = {}".format(year))
if where_clause:
where_clause_str = "WHERE " + " AND ".join(where_clause)
else:
where_clause_str = ""
c.execute("SELECT * FROM books {}".format(where_clause_str))
books = c.fetchall()
message = ""
if books:
for book in books:
message += "书名:{}\n作者:{}\n年份:{}\n状态:{}\n\n".format(book[1], book[2], book[3], book[4])
else:
message = "未找到符合条件的图书"
message_label.config(text=message)
search_button = Button(root, text="查询图书", command=search_books)
search_button.pack(pady=10)
# 借阅图书按钮
def borrow_book():
book_id = int(book_id_var.get())
borrower = borrower_var.get()
borrow_date = borrow_date_var.get()
return_date = return_date_var.get()
c.execute("SELECT status FROM books WHERE id = {}".format(book_id))
status = c.fetchone()[0]
if status == "在库":
c.execute("UPDATE books SET status = '借出' WHERE id = {}".format(book_id))
c.execute("INSERT INTO loans (book_id, borrower, borrow_date, return_date) VALUES (?, ?, ?, ?)",
(book_id, borrower, borrow_date, return_date))
conn.commit()
message_label.config(text="借阅成功")
else:
message_label.config(text="该图书已被借阅")
book_id_var = StringVar()
book_id_label = Label(root, text="图书编号:")
book_id_label.pack(pady=5)
book_id_entry = Entry(root, textvariable=book_id_var)
book_id_entry.pack()
borrower_var = StringVar()
borrower_label = Label(root, text="借阅人:")
borrower_label.pack(pady=5)
borrower_entry = Entry(root, textvariable=borrower_var)
borrower_entry.pack()
borrow_date_var = StringVar()
borrow_date_label = Label(root, text="借阅日期:")
borrow_date_label.pack(pady=5)
borrow_date_entry = Entry(root, textvariable=borrow_date_var)
borrow_date_entry.pack()
return_date_var = StringVar()
return_date_label = Label(root, text="归还日期:")
return_date_label.pack(pady=5)
return_date_entry = Entry(root, textvariable=return_date_var)
return_date_entry.pack()
borrow_button = Button(root, text="借阅图书", command=borrow_book)
borrow_button.pack(pady=10)
# 归还图书按钮
def return_book():
book_id = int(book_id_var.get())
c.execute("SELECT status FROM books WHERE id = {}".format(book_id))
status = c.fetchone()[0]
if status == "借出":
c.execute("UPDATE books SET status = '在库' WHERE id = {}".format(book_id))
c.execute("DELETE FROM loans WHERE book_id = {}".format(book_id))
conn.commit()
message_label.config(text="归还成功")
else:
message_label.config(text="该图书未被借阅")
return_button = Button(root, text="归还图书", command=return_book)
return_button.pack(pady=10)
# 关闭数据库连接
def close_db():
conn.close()
root.destroy()
root.protocol("WM_DELETE_WINDOW", close_db)
root.mainloop()
```
这个例子使用了 SQLite 数据库来存储图书和借阅信息。你可以根据自己的需要修改代码。
阅读全文
相关推荐
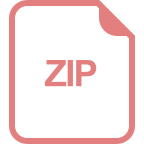
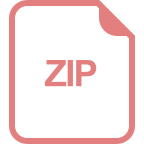
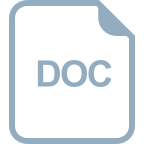
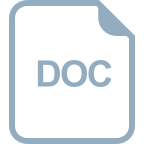
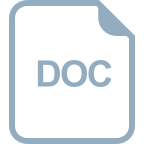
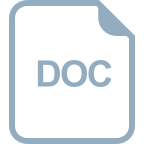
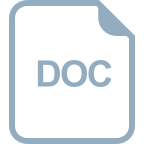
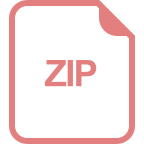
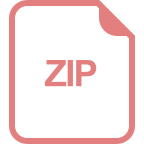
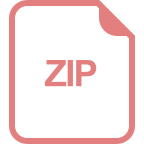
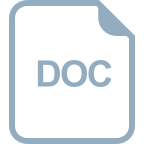
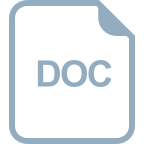

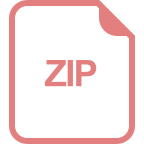
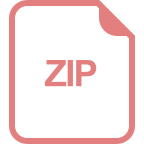
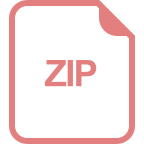

