定义一个基类有姓名、性别、年龄,再由基类派生出教师类和学生类。教师类增加工号、职称和工资,学生类增加学号、班级、专业和入学成绩。 编程要求: 1、设计虚函数输出教师和学生的基本信息; 2、计算教师的平均工资并输出; 3、使用友元函数重载运算符“<”,找出入学成绩最高的学生并输出;
时间: 2024-01-26 22:01:40 浏览: 78
好的,我可以帮你解答这个问题。以下是基类和派生类的定义及实现。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Person {
public:
Person(string n, char s, int a) : name(n), sex(s), age(a) {}
virtual void show() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "年龄:" << age << endl;
}
protected:
string name;
char sex;
int age;
};
class Teacher : public Person {
public:
Teacher(string n, char s, int a, int id, string t, double sal) : Person(n, s, a), id(id), title(t), salary(sal) {}
void show() override {
Person::show();
cout << "工号:" << id << endl;
cout << "职称:" << title << endl;
cout << "工资:" << salary << endl;
}
friend double AvgSalary(vector<Teacher>& teachers) {
double sum = 0.0;
for (auto& t : teachers) {
sum += t.salary;
}
return sum / teachers.size();
}
private:
int id;
string title;
double salary;
};
class Student : public Person {
public:
Student(string n, char s, int a, int id, string cl, string m, double g) : Person(n, s, a), id(id), cls(cl), major(m), grade(g) {}
void show() override {
Person::show();
cout << "学号:" << id << endl;
cout << "班级:" << cls << endl;
cout << "专业:" << major << endl;
cout << "成绩:" << grade << endl;
}
friend bool operator<(const Student& s1, const Student& s2) {
return s1.grade < s2.grade;
}
private:
int id;
string cls;
string major;
double grade;
};
int main() {
vector<Teacher> teachers = {
Teacher("张三", 'M', 35, 1001, "副教授", 8000),
Teacher("李四", 'F', 40, 1002, "教授", 10000),
Teacher("王五", 'M', 30, 1003, "讲师", 6000)
};
for (auto& t : teachers) {
t.show();
cout << endl;
}
cout << "平均工资:" << AvgSalary(teachers) << endl;
vector<Student> students = {
Student("小明", 'M', 18, 20180101, "计科1801", "计算机科学与技术", 89.5),
Student("小红", 'F', 19, 20180102, "计科1801", "计算机科学与技术", 92.0),
Student("小刚", 'M', 20, 20170101, "计科1701", "软件工程", 87.0)
};
auto it = max_element(begin(students), end(students));
it->show();
return 0;
}
```
上面的代码中,Person 是基类,Teacher 和 Student 是 Person 的派生类。在 Person 中定义了虚函数 show(),用于输出基本信息。在 Teacher 和 Student 中分别重写了 show() 函数,增加了各自特有的信息。注意,在 Teacher 中定义了友元函数 AvgSalary(),用于计算教师的平均工资。在 Student 中重载了小于号运算符,用于比较学生的入学成绩大小。
在 main() 函数中,首先定义了一个 vector 容器 teachers,其中存放了三个教师的信息,然后依次调用每个教师的 show() 函数输出信息,并调用 AvgSalary() 计算平均工资并输出。接着定义了一个 vector 容器 students,其中存放了三个学生的信息,然后使用 max_element() 函数找出入学成绩最高的学生,并调用其 show() 函数输出信息。
阅读全文
相关推荐
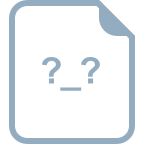
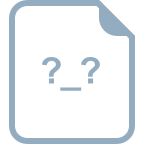
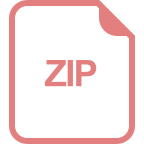








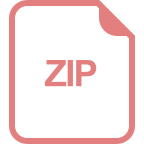
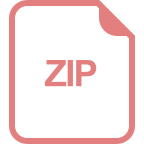
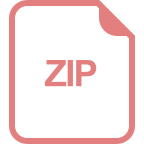
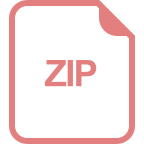