词频统计python代码
时间: 2023-07-09 11:40:00 浏览: 69
以下是一个简单的 Python 代码示例,可用于计算文本中每个单词的出现频率:
```python
text = "This is a sample text with several words. This is another sentence with some different words."
# 将文本分割成单词
words = text.split()
# 创建一个空字典来存储每个单词的出现次数
word_count = {}
# 遍历所有单词,并将它们添加到字典中
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
# 打印每个单词的出现次数
for word, count in word_count.items():
print(word, count)
```
此代码将文本分割成单词,并创建一个空字典来存储每个单词的出现次数。然后,它遍历所有单词,并将它们添加到字典中。最后,它打印每个单词的出现次数。
相关问题
词频统计 python 代码
在Python中,词频统计是一种常见的文本处理任务,通常用于分析文本数据中各个单词的出现频率。我们可以使用内置的collections模坓中的Counter类来快速完成这项工作。以下是一个简单的示例:
```python
from collections import Counter
def word_frequency(text):
# 将文本转换为小写并分割成单词列表
words = text.lower().split()
# 使用Counter计算每个单词的出现次数
frequency = Counter(words)
return frequency
# 示例文本
text = "这是一段示例文本,它包含了一些重复的词语,例如 示例 和 文本"
word_counts = word_frequency(text)
# 打印结果
for word, count in word_counts.most_common(): # .most_common()返回按频率降序排列的单词及其计数
print(f"{word}: {count}")
中文词频统计python代码
以下是一个简单的中文词频统计 Python 代码:
```python
import jieba
# 打开文件
with open('input.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 切分文本
words = jieba.cut(text)
# 计算词频
word_counts = {}
for word in words:
if len(word) > 1:
word_counts[word] = word_counts.get(word, 0) + 1
# 按词频排序
sorted_word_counts = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)
# 输出结果
for word, count in sorted_word_counts:
print(word, count)
```
解释:
1. 导入 jieba 库,用于中文分词。
2. 打开文件,并读取文本内容。
3. 利用 jieba.cut() 函数将文本切分成词语。
4. 遍历词语,统计每个词语出现的次数,保存在字典 word_counts 中。
5. 利用 sorted() 函数按照词频从大到小排序。
6. 遍历排序后的结果,输出每个词语和它的词频。
阅读全文
相关推荐
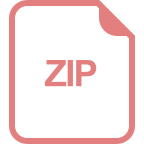
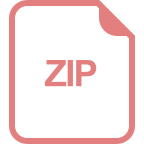












