pygame如何添加按钮
时间: 2023-09-07 14:11:28 浏览: 41
在Pygame中,可以通过创建一个矩形对象,并在矩形对象上显示文字来实现按钮的效果。具体来说,可以使用`pygame.draw.rect()`方法创建矩形,然后使用`pygame.draw.rect()`方法和`pygame.font.Font()`方法在矩形上显示文字。
下面是一个简单的示例代码,演示了如何在Pygame窗口中添加按钮:
```python
import pygame
pygame.init()
# 设置窗口大小和标题
window_size = (400, 400)
screen = pygame.display.set_mode(window_size)
pygame.display.set_caption("添加按钮示例")
# 设置字体和字号
font = pygame.font.Font(None, 36)
# 初始化按钮
button_rect = pygame.Rect(100, 100, 200, 50)
button_color = (255, 0, 0)
button_text = "Click Me!"
text_color = (255, 255, 255)
# 显示按钮
pygame.draw.rect(screen, button_color, button_rect)
text_surface = font.render(button_text, True, text_color)
text_rect = text_surface.get_rect(center=button_rect.center)
screen.blit(text_surface, text_rect)
pygame.display.flip()
# 等待用户关闭窗口
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 检测鼠标点击事件
if event.type == pygame.MOUSEBUTTONDOWN:
if button_rect.collidepoint(event.pos):
print("Button Clicked!")
# 更新窗口
pygame.display.update()
# 退出Pygame
pygame.quit()
```
在这个示例中,我们首先使用`pygame.Rect()`方法创建一个矩形对象,表示按钮的位置和大小,然后使用`pygame.draw.rect()`方法在窗口上绘制矩形,并使用`pygame.font.Font()`方法将文字渲染到一个Surface对象上,并将其添加到矩形中心的位置。然后,我们使用一个循环来等待用户点击按钮,一旦点击,就检测鼠标点击事件,并判断点击位置是否在按钮矩形内。
需要注意的是,在实际应用中,可能需要更复杂的逻辑来处理按钮的状态和事件,例如,使用一个类来表示按钮,并定义相关的方法和属性来处理按钮的状态和事件。
相关推荐
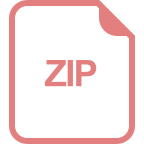














