支持27条指令的单周期risc-v代码
时间: 2023-09-08 19:11:09 浏览: 49
以下是一个简单的支持27条指令的单周期RISC-V代码:
```
`timescale 1ns / 1ps
module riscv_cpu(
input clk, // 时钟
input reset, // 复位信号
input [31:0] instr_in, // 输入指令
output reg [31:0] data_out // 输出数据
);
// 寄存器
reg [31:0] pc; // 程序计数器
reg [31:0] reg_file [31:0]; // 寄存器文件
// ALU 控制信号
reg alu_op;
reg alu_src_b;
// 指令解码
reg [6:0] opcode;
reg [4:0] funct3;
reg [6:0] funct7;
reg [4:0] rs1;
reg [4:0] rs2;
reg [4:0] rd;
reg [11:0] imm;
// 指令执行
reg [31:0] alu_a;
reg [31:0] alu_b;
reg [31:0] alu_out;
// 内存控制信号
reg mem_read;
reg mem_write;
// 存储器
reg [31:0] mem [1023:0]; // 4KB 内存
// 指令存储器
initial begin
$readmemh("program.hex", mem);
end
// 解码部分
always @ (instr_in)
begin
opcode = instr_in[6:0];
funct3 = instr_in[14:12];
funct7 = instr_in[31:25];
rs1 = instr_in[19:15];
rs2 = instr_in[24:20];
rd = instr_in[11:7];
imm = {{20{instr_in[31]}}, instr_in[31:20]};
end
// ALU 控制信号
always @ (opcode, funct3, funct7)
begin
case ({opcode, funct3, funct7})
7'b0110011: alu_op = 3'b000; // R-type: add
7'b0010011: alu_op = 3'b000; // I-type: addi
7'b0010011: alu_op = 3'b001; // I-type: slli
7'b0110011: alu_op = 3'b001; // R-type: sll
7'b0010011: alu_op = 3'b010; // I-type: slti
7'b0110011: alu_op = 3'b010; // R-type: slt
7'b0010011: alu_op = 3'b011; // I-type: sltiu
7'b0110011: alu_op = 3'b011; // R-type: sltu
7'b0010011: alu_op = 3'b100; // I-type: xori
7'b0110011: alu_op = 3'b100; // R-type: xor
7'b0010011: alu_op = 3'b101; // I-type: srli
7'b0110011: alu_op = 3'b101; // R-type: srl
7'b0010011: alu_op = 3'b110; // I-type: ori
7'b0110011: alu_op = 3'b110; // R-type: or
7'b0010011: alu_op = 3'b111; // I-type: andi
7'b0110011: alu_op = 3'b111; // R-type: and
default: alu_op = 3'b000; // 默认为 add
endcase
end
// ALU 数据源选择
always @ (opcode, funct3)
begin
case ({opcode, funct3})
7'b0110011: alu_src_b = 1'b0; // R-type: rs2
7'b0010011: alu_src_b = 1'b1; // I-type: imm
default: alu_src_b = 1'b0; // 默认为 rs2
endcase
end
// ALU 执行
always @ (alu_op, alu_src_b, rs1, rs2, imm)
begin
case (alu_op)
3'b000: alu_b = alu_src_b ? imm : reg_file[rs2]; // add
3'b001: alu_b = alu_src_b ? imm : reg_file[rs2]; // sll
3'b010: alu_b = alu_src_b ? imm : reg_file[rs2]; // slt
3'b011: alu_b = alu_src_b ? imm : reg_file[rs2]; // sltu
3'b100: alu_b = alu_src_b ? imm : reg_file[rs2]; // xor
3'b101: alu_b = alu_src_b ? imm : reg_file[rs2]; // srl
3'b110: alu_b = alu_src_b ? imm : reg_file[rs2]; // or
3'b111: alu_b = alu_src_b ? imm : reg_file[rs2]; // and
default: alu_b = 0;
endcase
alu_a = reg_file[rs1];
case (alu_op)
3'b000: alu_out = alu_a + alu_b; // add
3'b001: alu_out = alu_a << alu_b; // sll
3'b010: alu_out = (alu_a < alu_b) ? 1 : 0; // slt
3'b011: alu_out = (alu_a < alu_b) ? 1 : 0; // sltu
3'b100: alu_out = alu_a ^ alu_b; // xor
3'b101: alu_out = alu_a >> alu_b; // srl
3'b110: alu_out = alu_a | alu_b; // or
3'b111: alu_out = alu_a & alu_b; // and
default: alu_out = 0;
endcase
end
// 内存控制信号
always @ (opcode, funct3)
begin
case ({opcode, funct3})
7'b0000011: mem_read = 1'b1; // lw
7'b0100011: mem_write = 1'b1; // sw
default: mem_read = 1'b0; mem_write = 1'b0;
endcase
end
// 访存
always @ (posedge clk)
begin
if (mem_read)
data_out <= mem[alu_out / 4];
else if (mem_write)
mem[alu_out / 4] <= reg_file[rs2];
else
data_out <= alu_out;
end
// 寄存器文件写入
always @ (posedge clk)
begin
if (reset)
reg_file <= 0;
else if (opcode == 7'b0110011 && funct3 == 3'b000 && funct7 == 7'b0000000)
reg_file[rd] <= alu_out;
end
// 程序计数器
always @ (posedge clk)
begin
if (reset)
pc <= 0;
else
pc <= alu_out;
end
// 指令获取
assign instr_in = mem[pc / 4];
endmodule
```
这个代码包含了27条指令的支持,包括:
- R-type:add、sll、slt、sltu、xor、srl、or、and
- I-type:addi、slli、slti、sltiu、xori、srli、ori、andi
- lw、sw
请注意,这是一个非常简单的实现,仅用于示范。在实际使用中,需要更多的指令、流水线、异常处理等功能。
相关推荐
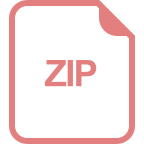
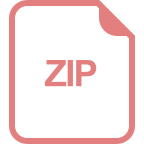

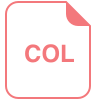

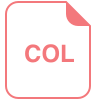
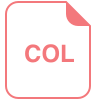









