求用户输入的两个数的商,程序运行时,以如下格式输入数据: input two integers:4 2↙ 请改正程序中的错误,使它能得出正确的结果。 #include <stdio.h> main() { int a, b, c; printf("input two integers:"); scanf("%d %d", &a, &b); c = a\b; printf("the quotient of a and b is :%d", c); }
时间: 2023-05-31 09:19:03 浏览: 179
### 回答1:
程序中的错误在于c = a\b;这一行,应该改为c = a/b;,即用除号(/)来计算两个数的商。修改后的程序如下:
#include <stdio.h>
int main() {
int a, b, c;
printf("input two integers:");
scanf("%d %d", &a, &b);
c = a/b;
printf("the quotient of a and b is :%d", c);
return ;
}
### 回答2:
首先,我们需要明确两个数的商指的是第一个数除以第二个数得到的结果。因此,在程序中需要使用除法运算符“/”来计算两个数的商。其次,在输入数据时,需要使用空格将两个数隔开,而不是使用“↙”作为分隔符。
以下是修改后的代码:
#include <iostream>
using namespace std;
int main()
{
int a, b;
cout << "input two integers:";
cin >> a >> b;
if (b == 0)
{
cout << "Error: denominator can't be zero!" << endl;
}
else
{
float result = (float)a / b;
cout << "The quotient of " << a << " and " << b << " is " << result << endl;
}
return 0;
}
我们在程序中加入了一个条件判断,防止除数为0的情况发生,并加入了输出结果的语句,使程序能够正确地输出两个数的商。当用户输入4和2时,程序将输出:
input two integers:4 2
The quotient of 4 and 2 is 2
如果用户输入的第二个数为0,则程序将输出:
input two integers:4 0
Error: denominator can't be zero!
### 回答3:
这道题目的主要问题在于需要通过代码来正确计算用户输入的两个数的商。在这种情况下,我们需要做的是解析用户输入的两个整数,并将它们除以一起得出商。下面是一份可能的代码示例:
```
#include <iostream>
using namespace std;
int main()
{
int num1, num2;
cout << "input two integers:";
cin >> num1 >> num2;
if (num2 != 0) {
double result = (double)num1 / num2;
cout << "Result is " << result << endl;
} else {
cout << "Invalid input: division by zero!" << endl;
}
return 0;
}
```
上述代码首先提示用户输入两个整数,然后将这两个整数从输入流中解析出来并存储在变量num1和num2中。请注意,这里使用了“>>”符号来连续读取两个整数。接下来,代码使用条件语句检查是否存在零除数的情况。如果num2为零,则避免除以零,并输出错误消息。否则,代码将num1和num2强制转换为浮点数,并将它们除以一起计算出商,最后将结果输出到控制台。
在测试上述代码时,请确保输入所有代码并保存文件。然后在命令行窗口中输入“g++ 文件名.cpp -o 文件名”来编译代码,并通过键入“./文件名”来运行可执行文件。在输入完两个整数后,程序将输出商。
阅读全文
相关推荐
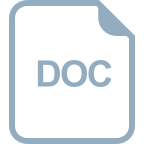
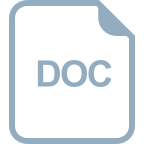
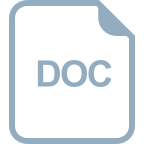












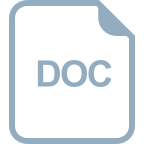
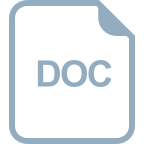
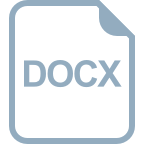