读取存储《哈姆雷特》英文剧本的文件,分析统计其中单词出现的频率,使用turtle模块绘制词频统计结果,以柱状图的形式展示统计结果。
时间: 2024-02-16 08:03:04 浏览: 55
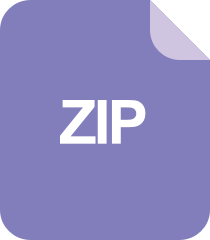
英文文本单词词频统计(C++实现)

好的,我会尽力回答你的问题。
首先需要读取《哈姆雷特》英文剧本的文件,可以使用Python中的open函数打开文件,并使用read方法读取文件内容。代码如下:
```python
with open("hamlet.txt", "r") as f:
text = f.read()
```
接下来,我们需要对文本进行预处理,去除标点符号、转换为小写等,以便后续统计。可以使用Python中的re模块和string模块来实现。代码如下:
```python
import re
import string
# 去除标点符号和数字
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\d+', '', text)
# 转换为小写
text = text.lower()
# 去除换行符
text = text.replace('\n', ' ')
# 将文本分割为单词列表
words = text.split()
```
接下来,使用Python中的collections模块中的Counter类来统计单词出现的频率。代码如下:
```python
from collections import Counter
word_counts = Counter(words)
```
最后,使用turtle模块绘制词频统计结果,以柱状图的形式展示统计结果。代码如下:
```python
import turtle
# 设置画布大小和起始位置
turtle.setup(800, 600)
turtle.penup()
turtle.goto(-350, -250)
# 设置柱状图参数
bar_width = 20
bar_gap = 10
max_height = 400
# 遍历单词列表,绘制柱状图
for i, (word, count) in enumerate(word_counts.most_common(20)):
turtle.pendown()
turtle.setheading(90)
turtle.forward(count / max(word_counts.values()) * max_height)
turtle.write(f"{word}: {count}", align="center", font=("Arial", 10, "normal"))
turtle.right(90)
turtle.forward(bar_width)
turtle.right(90)
turtle.forward(count / max(word_counts.values()) * max_height)
turtle.penup()
turtle.goto(-350 + (bar_width + bar_gap) * (i + 1), -250)
```
运行以上代码,即可得到绘制好的柱状图。注意,需要提前安装turtle模块。
阅读全文
相关推荐
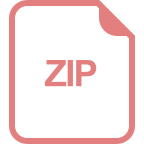



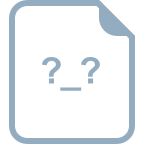
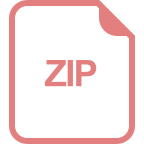
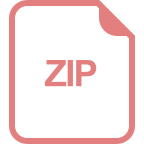
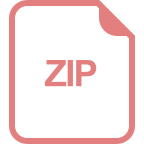
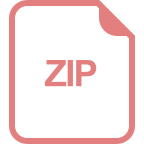







