写一段代码使用系统调用shmget()、shmat()、msgmdt()、shmctl(),实现Server和 Client进程之间的通信。
时间: 2024-05-03 21:20:49 浏览: 125
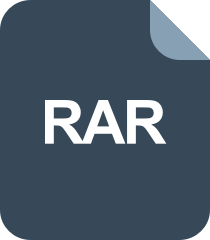
服务器和客户端通信

好的,下面是实现Server和Client进程之间通信的代码:
Server进程:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <sys/msg.h>
#include <string.h>
#define SHM_SIZE 1024
int main() {
int shmid;
char *shmaddr;
key_t key = ftok(".", 'a'); // 根据当前目录和'a'生成key值
// 创建共享内存
shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666);
if (shmid < 0) {
perror("shmget error");
exit(1);
}
// 映射共享内存到进程空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat error");
exit(1);
}
// 发送消息
strcpy(shmaddr, "Hello, client!");
// 调用shmctl函数删除共享内存
if (shmctl(shmid, IPC_RMID, 0) < 0) {
perror("shmctl error");
exit(1);
}
// 解除映射
shmdt(shmaddr);
printf("Server exit successfully.\n");
return 0;
}
```
Client进程:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <sys/msg.h>
#include <string.h>
#define SHM_SIZE 1024
int main() {
int shmid;
char *shmaddr;
key_t key = ftok(".", 'a'); // 根据当前目录和'a'生成key值
// 获取共享内存
shmid = shmget(key, SHM_SIZE, 0666);
if (shmid < 0) {
perror("shmget error");
exit(1);
}
// 映射共享内存到进程空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat error");
exit(1);
}
// 接收消息
printf("Server says: %s\n", shmaddr);
// 解除映射
shmdt(shmaddr);
printf("Client exit successfully.\n");
return 0;
}
```
阅读全文
相关推荐
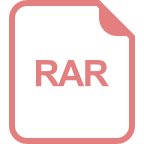

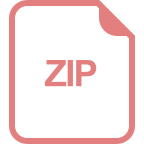
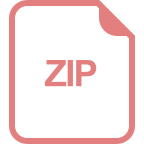
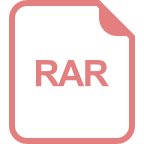
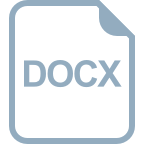
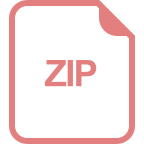
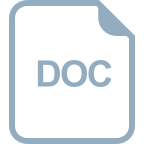
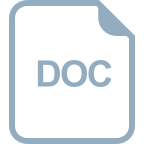
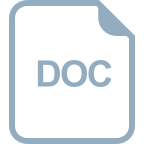
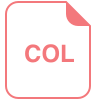
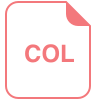
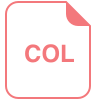
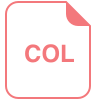
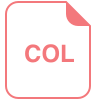
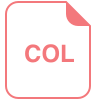
