字符串实验报告
时间: 2023-07-25 21:08:37 浏览: 106
实验名称:字符串操作实验
实验目的:通过实现字符串的常见操作,掌握字符串的基本概念和操作方式。
实验环境:C++语言,Visual Studio 2019
实验步骤:
1. 字符串定义和初始化
在C++中,字符串可以用字符数组或string类型来表示。字符数组定义方式如下:
```
char str[20]; //定义一个长度为20的字符数组
```
string类型定义方式如下:
```
string s; //定义一个空字符串
```
字符串初始化可以直接在定义时赋值,也可以通过赋值语句进行赋值。例如:
```
char str1[] = "hello world"; //定义并初始化字符数组
string s1 = "hello world"; //定义并初始化string类型
char str2[20];
strcpy(str2, "hello world"); //使用strcpy函数进行字符串复制
```
2. 字符串输入输出
可以使用cin和cout语句进行字符串的输入和输出。对于字符数组,需要使用gets和puts函数进行输入输出。例如:
```
char str[20];
cout << "请输入字符串:";
gets(str); //使用gets函数进行字符串输入
cout << "你输入的字符串是:" << str << endl; //使用puts函数进行字符串输出
```
对于string类型的字符串,可以直接使用cin和cout语句进行输入输出。例如:
```
string s;
cout << "请输入字符串:";
cin >> s; //使用cin语句进行字符串输入
cout << "你输入的字符串是:" << s << endl; //使用cout语句进行字符串输出
```
3. 字符串长度
可以使用strlen函数获取字符数组的长度,也可以使用string类型的size函数获取字符串的长度。例如:
```
char str[] = "hello world";
int len1 = strlen(str); //获取字符数组的长度
string s = "hello world";
int len2 = s.size(); //获取字符串的长度
```
4. 字符串连接
可以使用strcat函数将两个字符数组连接起来,也可以使用+运算符将两个string类型的字符串连接起来。例如:
```
char str1[] = "hello";
char str2[] = "world";
char str3[20] = "";
strcat(str3, str1); //将str1复制到str3中
strcat(str3, str2); //将str2连接到str3中
cout << str3 << endl; //输出str3
string s1 = "hello";
string s2 = "world";
string s3 = s1 + s2; //将s1和s2连接到一起
cout << s3 << endl; //输出s3
```
5. 字符串比较
可以使用strcmp函数比较两个字符数组是否相等,也可以使用==运算符比较两个string类型的字符串是否相等。例如:
```
char str1[] = "hello";
char str2[] = "world";
if (strcmp(str1, str2) == 0) {
cout << "str1和str2相等" << endl;
} else {
cout << "str1和str2不相等" << endl;
}
string s1 = "hello";
string s2 = "world";
if (s1 == s2) {
cout << "s1和s2相等" << endl;
} else {
cout << "s1和s2不相等" << endl;
}
```
实验结果:
根据以上步骤,我们可以实现字符串的常见操作,包括定义和初始化、输入输出、长度获取、连接和比较等。通过实验,我掌握了字符串的基本概念和操作方式,提升了自己的编程能力。
相关推荐
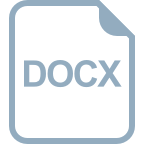
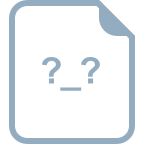














