c++子字符串出现次数
时间: 2023-08-24 07:06:01 浏览: 111
要统计一个字符串中某个子字符串出现的次数,可以使用字符串匹配的方法。比较常见的方法有暴力匹配、KMP算法、Boyer-Moore算法等。
下面是暴力匹配的代码实现:
```c
#include <stdio.h>
#include <string.h>
int countSubstr(char *str, char *sub)
{
int count = 0;
int len1 = strlen(str);
int len2 = strlen(sub);
for (int i = 0; i <= len1 - len2; i++)
{
int j;
for (j = 0; j < len2; j++)
{
if (str[i+j] != sub[j])
break;
}
if (j == len2)
count++;
}
return count;
}
int main()
{
char str[] = "hello world, world is beautiful";
char sub[] = "world";
int count = countSubstr(str, sub);
printf("'%s' appears %d times in '%s'\n", sub, count, str);
return 0;
}
```
输出结果为:
```
'world' appears 2 times in 'hello world, world is beautiful'
```
这里的实现比较简单,时间复杂度为 O(n*m),其中 n 和 m 分别为原字符串和子字符串的长度。如果需要更高效的实现,可以考虑使用 KMP 算法或 Boyer-Moore 算法。
阅读全文
相关推荐
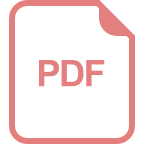

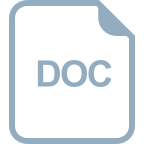










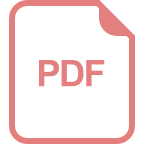
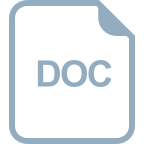
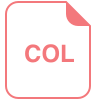
