unity Vector3d 具体代码
时间: 2023-07-03 17:11:16 浏览: 50
在 Unity 中使用 Vector3D,可以使用 Vector3 类型,Vector3 包含三个浮点数,分别代表 x、y、z 坐标轴上的值。
下面是一个示例代码:
```
using UnityEngine;
public class Example : MonoBehaviour
{
void Start()
{
// 创建一个 Vector3 对象,代表在 x、y、z 上的偏移量为 (1, 2, 3)
Vector3 offset = new Vector3(1, 2, 3);
// 获取当前物体的坐标
Vector3 position = transform.position;
// 将当前物体的坐标与偏移量相加,得到新的位置
Vector3 newPosition = position + offset;
// 将物体移动到新的位置
transform.position = newPosition;
}
}
```
在这个示例中,我们创建了一个 Vector3 对象,代表在 x、y、z 上的偏移量为 (1, 2, 3),然后获取当前物体的坐标,将其与偏移量相加,得到新的位置,最后将物体移动到新的位置。
相关问题
Vector3d 代码
实际上,在 Unity 中使用的是 Vector3 而不是 Vector3D,它们的用法和定义略有不同。下面是 Vector3 的 C# 代码示例:
```c#
using UnityEngine;
public class Example : MonoBehaviour
{
void Start()
{
// 创建一个 Vector3 对象,代表在 x、y、z 上的偏移量为 (1, 2, 3)
Vector3 offset = new Vector3(1, 2, 3);
// 获取当前物体的坐标
Vector3 position = transform.position;
// 将当前物体的坐标与偏移量相加,得到新的位置
Vector3 newPosition = position + offset;
// 将物体移动到新的位置
transform.position = newPosition;
}
}
```
在这个示例中,我们创建了一个 Vector3 对象,代表在 x、y、z 上的偏移量为 (1, 2, 3),然后获取当前物体的坐标,将其与偏移量相加,得到新的位置,最后将物体移动到新的位置。
unity3d跳跃代码
Unity3D中实现角色跳跃功能通常有两种方式:使用刚体组件或使用Character Controller组件。以下是使用刚体组件实现跳跃的示例代码:
```
using UnityEngine;
public class Jump : MonoBehaviour
{
public float jumpForce = 10f; //跳跃力度
public Transform feetPos; //脚底位置,用于检测是否在地面上
public float checkRadius = 0.5f; //检测半径
public LayerMask whatIsGround; //定义地面的Layer
private Rigidbody2D rb;
private bool isGrounded; //是否在地面上
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
isGrounded = Physics2D.OverlapCircle(feetPos.position, checkRadius, whatIsGround); //检测是否在地面上
if (isGrounded && Input.GetKeyDown(KeyCode.Space)) //如果在地面上并按下跳跃键
{
rb.velocity = Vector2.up * jumpForce; //给刚体施加向上的力
}
}
}
```
相关问题:
1. 什么是刚体组件?
2. 什么是Character Controller组件?
3. 如何检测角色是否在地面上?
4. 如何给刚体组件施加力?
相关推荐
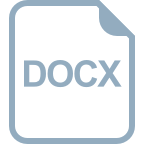
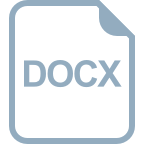
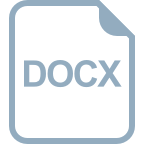
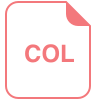
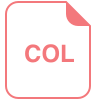
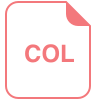
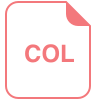
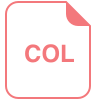







