linux编写代码,实现两个线程互斥运行,要求线程一完整输出1-50,线程二完整输出51-100,必须保证线程一,线程二的完整执行.
时间: 2024-02-06 22:09:13 浏览: 130
这里提供一种使用互斥锁(mutex)实现的方法,具体代码如下:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex; // 定义互斥锁
int count = 0; // 全局变量
void *thread1(void *arg) {
for (int i = 1; i <= 50; i++) {
pthread_mutex_lock(&mutex); // 申请互斥锁
printf("Thread 1: %d\n", count++);
pthread_mutex_unlock(&mutex); // 释放互斥锁
}
}
void *thread2(void *arg) {
for (int i = 51; i <= 100; i++) {
pthread_mutex_lock(&mutex); // 申请互斥锁
printf("Thread 2: %d\n", count++);
pthread_mutex_unlock(&mutex); // 释放互斥锁
}
}
int main() {
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL); // 初始化互斥锁
pthread_create(&tid1, NULL, thread1, NULL);
pthread_create(&tid2, NULL, thread2, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex); // 销毁互斥锁
return 0;
}
```
首先在主函数中初始化互斥锁,然后通过 `pthread_create` 创建两个线程,分别执行 `thread1` 和 `thread2` 函数。在这两个函数中,使用 `pthread_mutex_lock` 申请互斥锁并输出相应的数字,然后使用 `pthread_mutex_unlock` 释放互斥锁。最后在主函数中等待两个线程执行完毕并销毁互斥锁。
这样,就能保证线程一和线程二的完整执行,并且不会出现竞争条件。
阅读全文
相关推荐
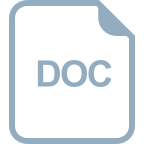
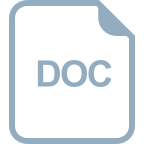
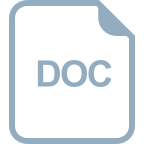
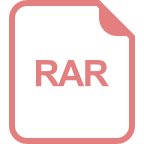
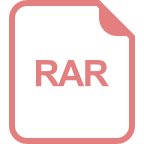
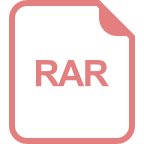
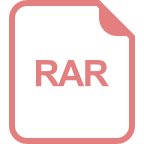
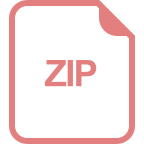
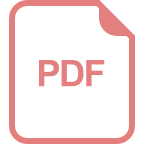
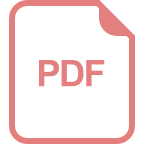
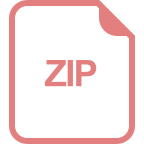
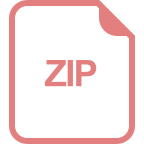
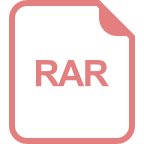
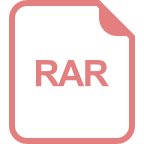
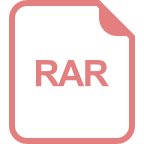
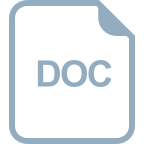
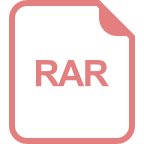
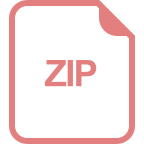
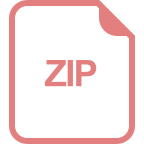