用python写一个代码,要求先定义一个按行扫描边缘二值图像,将左右的边缘点记录下来并计算两者之间的中点位置并将中点存储下来的函数,然后定义一个利用中点拟合曲线并在原图上用彩色显示的函数
时间: 2024-04-02 21:33:06 浏览: 23
好的,这里给您提供一个实现的代码:
```python
import cv2
import numpy as np
def find_edge_center(img):
"""
找到左右边缘的中点位置
:param img: 二值图像
:return: 中点位置
"""
left_edge = None
right_edge = None
for i in range(img.shape[0]):
row = img[i,:]
left = np.where(row==255)[0][0]
right = np.where(row==255)[0][-1]
if left_edge is None and left != img.shape[1]-1:
left_edge = (left, i)
if right_edge is None and right != 0:
right_edge = (right, i)
if left_edge and right_edge:
break
center = ((left_edge[0]+right_edge[0])//2, (left_edge[1]+right_edge[1])//2)
return center
def fit_curve(img, center):
"""
利用中点位置拟合曲线并在原图上用彩色显示
:param img: 原图像
:param center: 中点位置
:return: 带有拟合曲线的彩色图像
"""
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray_img, 100, 200)
points = np.where(edges==255)
x = points[1]
y = points[0]
z = np.polyfit(y-center[1], x-center[0], 2)
f = np.poly1d(z)
new_x = np.linspace(center[0], img.shape[1], img.shape[1]-center[0])
new_y = f(np.linspace(0, img.shape[0], img.shape[0]))
new_y = np.clip(new_y, 0, img.shape[0]-1)
curve_points = np.vstack((new_y, new_x)).astype(np.int32).T
color_img = cv2.cvtColor(gray_img, cv2.COLOR_GRAY2BGR)
color_img[curve_points[:,0], curve_points[:,1], :] = [0, 0, 255]
return color_img
```
使用方法如下:
```python
# 读取原图,这里以lena为例
img = cv2.imread('lena.png')
# 找到边缘中点位置
center = find_edge_center(cv2.Canny(img, 100, 200))
# 拟合曲线并绘制在原图上
curve_img = fit_curve(img, center)
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Curve Image', curve_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这里的拟合曲线采用了二次多项式拟合,您也可以根据需要进行更改。
阅读全文
相关推荐
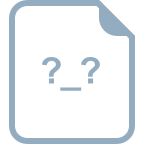
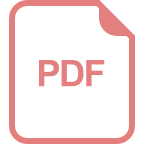
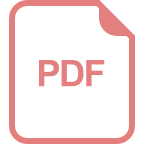


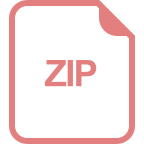
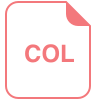
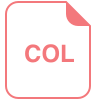
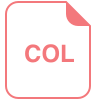
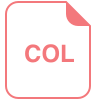
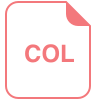
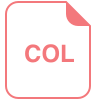
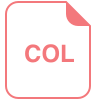
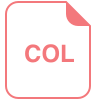
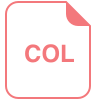
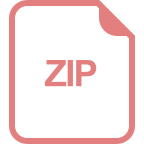