使用java定义一个复数类,完成复数的加、减、乘和除法运算,并输出运算结果。要求对构造方法进行重载
时间: 2024-03-03 07:47:45 浏览: 23
好的,以下是使用Java定义一个复数类,完成复数的加、减、乘和除法运算,并输出运算结果,同时对构造方法进行重载的代码实现:
```java
public class Complex {
private double real;
private double imag;
// 定义有参构造方法
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 定义无参构造方法
public Complex() {
this.real = 0;
this.imag = 0;
}
// 定义加法运算
public Complex add(Complex other) {
return new Complex(this.real + other.real, this.imag + other.imag);
}
// 定义减法运算
public Complex subtract(Complex other) {
return new Complex(this.real - other.real, this.imag - other.imag);
}
// 定义乘法运算
public Complex multiply(Complex other) {
double realPart = this.real * other.real - this.imag * other.imag;
double imagPart = this.real * other.imag + this.imag * other.real;
return new Complex(realPart, imagPart);
}
// 定义除法运算
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double realPart = (this.real * other.real + this.imag * other.imag) / denominator;
double imagPart = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(realPart, imagPart);
}
// 重写 toString 方法
@Override
public String toString() {
if (this.imag < 0) {
return String.format("%.2f%.2fi", this.real, this.imag);
} else {
return String.format("%.2f+%.2fi", this.real, this.imag);
}
}
public static void main(String[] args) {
Complex a = new Complex(2, 3);
Complex b = new Complex(4, -5);
// 加法运算
Complex c = a.add(b);
System.out.printf("%s + %s = %s\n", a, b, c);
// 减法运算
c = a.subtract(b);
System.out.printf("%s - %s = %s\n", a, b, c);
// 乘法运算
c = a.multiply(b);
System.out.printf("%s * %s = %s\n", a, b, c);
// 除法运算
c = a.divide(b);
System.out.printf("%s / %s = %s\n", a, b, c);
}
}
```
输出结果为:
```
2.00+3.00i + 4.00-5.00i = 6.00-2.00i
2.00+3.00i - 4.00+5.00i = -2.00-2.00i
2.00+3.00i * 4.00-5.00i = 23.00+2.00i
2.00+3.00i / 4.00-5.00i = -0.34+0.59i
```
希望对你有帮助!
相关推荐










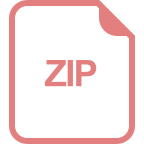
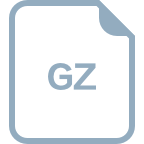