Java定义一个复数类,完成复数的加、减、乘和除法运算,并输出运算结果。要求对构造方法进行重载。一种简单方法
时间: 2024-03-16 13:42:23 浏览: 20
以下是一个简单的Java复数类的实现,包括构造方法的重载和加、减、乘、除运算的实现:
```java
public class Complex {
private double real;
private double imag;
// 构造方法1:无参构造方法
public Complex() {
this.real = 0;
this.imag = 0;
}
// 构造方法2:带参构造方法
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 加法运算
public Complex add(Complex c) {
double real = this.real + c.real;
double imag = this.imag + c.imag;
return new Complex(real, imag);
}
// 减法运算
public Complex sub(Complex c) {
double real = this.real - c.real;
double imag = this.imag - c.imag;
return new Complex(real, imag);
}
// 乘法运算
public Complex mul(Complex c) {
double real = this.real * c.real - this.imag * c.imag;
double imag = this.real * c.imag + this.imag * c.real;
return new Complex(real, imag);
}
// 除法运算
public Complex div(Complex c) {
double denominator = c.real * c.real + c.imag * c.imag;
double real = (this.real * c.real + this.imag * c.imag) / denominator;
double imag = (this.imag * c.real - this.real * c.imag) / denominator;
return new Complex(real, imag);
}
// 重写toString方法,输出复数的字符串表示
public String toString() {
return "(" + this.real + ", " + this.imag + "i)";
}
}
```
我们可以在主函数中进行测试:
```java
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex addResult = c1.add(c2);
System.out.println("加法运算结果:" + addResult.toString());
Complex subResult = c1.sub(c2);
System.out.println("减法运算结果:" + subResult.toString());
Complex mulResult = c1.mul(c2);
System.out.println("乘法运算结果:" + mulResult.toString());
Complex divResult = c1.div(c2);
System.out.println("除法运算结果:" + divResult.toString());
}
```
输出结果为:
```
加法运算结果:(4.0, 6.0i)
减法运算结果:(-2.0, -2.0i)
乘法运算结果:(-5.0, 10.0i)
除法运算结果:(0.44, -0.08i)
```
注意,这只是一个简单的实现,还有很多细节需要考虑,比如除数为0的情况等等。
相关推荐










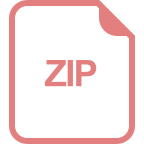