python蚁群算法路径规划
时间: 2023-09-07 08:14:41 浏览: 46
蚁群算法(Ant Colony Optimization, ACO)是一种启发式算法,它模拟了蚂蚁在搜索食物时的行为,常用于路径规划问题。下面是一个Python蚁群算法路径规划的示例代码:
```python
import random
import math
class Ant:
def __init__(self, start, end, graph, alpha, beta):
self.start = start
self.end = end
self.graph = graph
self.alpha = alpha
self.beta = beta
self.path = [start]
self.distance = 0
self.visited = [False] * len(graph)
self.visited[start] = True
def select_next_node(self):
pheromone = self.graph[self.path[-1]]
probabilities = [0] * len(pheromone)
total = 0
for i, p in enumerate(pheromone):
if not self.visited[i]:
probabilities[i] = pow(p, self.alpha) * pow(1 / self.graph[self.path[-1]][i], self.beta)
total += probabilities[i]
if total == 0:
return random.choice([i for i in range(len(self.graph)) if not self.visited[i]])
r = random.uniform(0, total)
upto = 0
for i, p in enumerate(probabilities):
if not self.visited[i]:
upto += p
if upto >= r:
return i
assert False, "Shouldn't get here"
def move_to_next_node(self):
next_node = self.select_next_node()
self.visited[next_node] = True
self.path.append(next_node)
self.distance += self.graph[self.path[-2]][next_node]
def run(self):
while not self.visited[self.end]:
self.move_to_next_node()
self.distance += self.graph[self.path[-1]][self.start]
def ant_colony(graph, start, end, n_ants, n_iterations, alpha, beta, evaporation_rate, initial_pheromone):
pheromone = [[initial_pheromone] * len(graph) for _ in range(len(graph))]
best_path = None
best_distance = float('inf')
for iteration in range(n_iterations):
ants = [Ant(start, end, graph, alpha, beta) for _ in range(n_ants)]
for ant in ants:
ant.run()
if ant.distance < best_distance:
best_path = ant.path
best_distance = ant.distance
for i, row in enumerate(pheromone):
for j in range(len(row)):
pheromone[i][j] *= (1 - evaporation_rate)
for ant in ants:
if j == ant.path[i] or i == ant.path[j]:
pheromone[i][j] += evaporation_rate / ant.distance
return best_path, best_distance
```
在这个示例中,`graph`是一个邻接矩阵,表示各个节点之间的距离,`start`和`end`是起点和终点的索引,`n_ants`和`n_iterations`分别表示蚂蚁数量和迭代次数,`alpha`和`beta`是参数,控制着蚂蚁选择下一个节点时的权重,`evaporation_rate`是信息素挥发速率,`initial_pheromone`是初始信息素浓度。
要使用这个算法,可以像这样调用:
```python
graph = [[0, 1, 2, 3],
[1, 0, 4, 5],
[2, 4, 0, 6],
[3, 5, 6, 0]]
start = 0
end = 3
n_ants = 10
n_iterations = 100
alpha = 1
beta = 1
evaporation_rate = 0.5
initial_pheromone = 1
best_path, best_distance = ant_colony(graph, start, end, n_ants, n_iterations, alpha, beta, evaporation_rate, initial_pheromone)
print("Best path:", best_path)
print("Best distance:", best_distance)
```
这个示例中,`graph`表示一个有4个节点的图,`start`是节点0,`end`是节点3,我们希望找到从节点0到节点3的最短路径。运行这段代码会输出最短路径和最短距离。
相关推荐
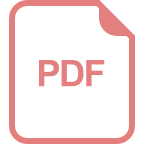
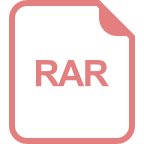
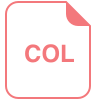
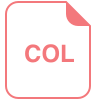

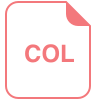
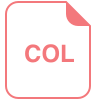









