蚁群算法 路径规划 python
时间: 2023-10-09 09:09:52 浏览: 76
蚁群算法是一种模仿蚂蚁觅食行为的启发式优化算法,主要用于解决路径规划问题。它通过模拟蚂蚁在寻找食物时的行为,不断地在问题空间中搜索并更新路径,最终找到一条较优的解决方案。
在Python中,可以使用一些库来实现蚁群算法的路径规划。以下是一个简单的示例代码:
```python
import numpy as np
class AntColony:
def __init__(self, num_ants, num_iterations, num_cities, alpha=1, beta=5, rho=0.5, Q=100):
self.num_ants = num_ants
self.num_iterations = num_iterations
self.num_cities = num_cities
self.alpha = alpha
self.beta = beta
self.rho = rho
self.Q = Q
self.distances = np.array([[0, 2, 3, 0],
[2, 0, 1, 4],
[3, 1, 0, 2],
[0, 4, 2, 0]]) # 城市之间的距离
self.pheromone = np.ones((num_cities, num_cities)) # 信息素矩阵
def run(self):
best_path = None
best_length = np.inf
for i in range(self.num_iterations):
all_paths = []
all_lengths = []
for ant in range(self.num_ants):
path = self.construct_path()
length = self.calculate_path_length(path)
if length < best_length:
best_length = length
best_path = path
all_paths.append(path)
all_lengths.append(length)
self.update_pheromone(all_paths, all_lengths)
return best_path, best_length
def construct_path(self):
path = [0]
visited = set([0])
for _ in range(self.num_cities - 1):
next_city = self.next_city(path[-1], visited)
path.append(next_city)
visited.add(next_city)
return path
def next_city(self, current_city, visited):
unvisited = list(set(range(self.num_cities)) - visited)
probabilities = []
for city in unvisited:
pheromone = self.pheromone[current_city][city]
distance = self.distances[current_city][city]
probability = pheromone**self.alpha / distance**self.beta
probabilities.append(probability)
probabilities = np.array(probabilities)
probabilities = probabilities / probabilities.sum()
next_city = np.random.choice(unvisited, p=probabilities)
return next_city
def calculate_path_length(self, path):
length = 0
for i in range(len(path) - 1):
length += self.distances[path[i]][path[i + 1]]
return length
def update_pheromone(self, all_paths, all_lengths):
self.pheromone *= (1 - self.rho)
for i in range(len(all_paths)):
path = all_paths[i]
length = all_lengths[i]
for j in range(len(path) - 1):
city_a = path[j]
city_b = path[j + 1]
self.pheromone[city_a][city_b] += self.Q / length
if __name__ == '__main__':
ant_colony = AntColony(num_ants=10, num_iterations=100, num_cities=4)
best_path, best_length = ant_colony.run()
print("Best path:", best_path)
print("Best length:", best_length)
```
在上述代码中,我们使用了一个4个城市的路径规划问题作为示例,距离矩阵和信息素矩阵分别用`distances`和`pheromone`表示。你可以根据自己的实际问题进行修改和扩展。
希望对你有帮助!如果有任何疑问,请随时提问。
相关推荐
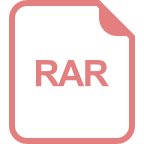
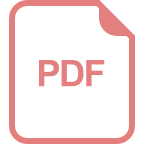














