蚁群算法python路径规划
时间: 2023-09-07 11:14:47 浏览: 52
蚁群算法(Ant Colony Optimization,简称ACO)是一种基于蚂蚁群体智能的启发式搜索算法,常用于求解路径规划问题。下面给出一个简单的Python实现,用于解决一个简单的路径规划问题。
首先,我们需要定义一个问题:假设有一个二维的迷宫,其中包含起点和终点以及一些障碍物,我们需要找到从起点到终点的最短路径,同时要避开障碍物。我们可以将迷宫表示为一个二维数组,其中0表示可通行的空间,1表示障碍物。
```python
import numpy as np
# 定义迷宫,0表示可通行的空间,1表示障碍物
maze = np.array([
[0, 0, 0, 0, 0, 0, 0, 1],
[0, 1, 1, 1, 0, 1, 0, 1],
[0, 0, 0, 0, 0, 0, 0, 1],
[0, 1, 0, 1, 1, 1, 0, 0],
[0, 1, 0, 0, 0, 0, 1, 0],
[0, 1, 1, 1, 0, 1, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 1],
[0, 1, 1, 1, 0, 1, 0, 0]
])
# 定义起点和终点
start = (0, 0)
end = (7, 7)
```
接下来,我们需要定义一个蚂蚁类,用于实现蚂蚁的移动和路径更新:
```python
class Ant:
def __init__(self, start, end, maze):
self.position = start
self.path = [start]
self.end = end
self.maze = maze
def move(self, alpha=1, beta=2, Q=100, pheromone=0.1):
while self.position != self.end:
row, col = self.position
neighbors = []
# 获取周围可通行的位置
for i in range(row-1, row+2):
for j in range(col-1, col+2):
if i >= 0 and j >= 0 and i < len(maze) and j < len(maze[0]) and maze[i][j] == 0 and (i, j) not in self.path:
neighbors.append((i, j))
# 计算每个邻居的概率
probabilities = []
for neighbor in neighbors:
ph = pheromone ** alpha
distance = ((neighbor[0] - self.end[0]) ** 2 + (neighbor[1] - self.end[1]) ** 2) ** 0.5
heuristic = distance ** beta
probabilities.append(ph * heuristic)
probabilities = np.array(probabilities)
probabilities /= probabilities.sum()
# 根据概率选择下一个位置
next_position = neighbors[np.random.choice(len(neighbors), p=probabilities)]
self.path.append(next_position)
self.position = next_position
# 更新路径上的信息素
for i in range(len(self.path)-1):
row1, col1 = self.path[i]
row2, col2 = self.path[i+1]
maze[row1][col1] += Q / ((row2-row1)**2 + (col2-col1)**2)
```
在蚂蚁类中,我们首先定义了蚂蚁的起点、终点、当前位置和已经走过的路径。然后,我们定义了一个move方法,用于实现蚂蚁的移动和路径更新。在move方法中,我们首先获取当前位置周围可通行的位置,然后计算每个邻居的概率,根据概率选择下一个位置。在选择下一个位置后,我们更新蚂蚁的位置和已经走过的路径。最后,我们根据路径上的信息素,更新迷宫中的信息素。
接下来,我们可以使用多个蚂蚁来搜索最短路径:
```python
# 初始化信息素
pheromone = np.ones_like(maze) * 0.1
# 定义参数
alpha = 1
beta = 2
Q = 100
n_ants = 100
n_iterations = 100
# 开始搜索
for iteration in range(n_iterations):
ants = [Ant(start, end, maze) for _ in range(n_ants)]
for ant in ants:
ant.move(alpha, beta, Q, pheromone)
# 更新信息素
pheromone *= 0.9
for ant in ants:
for i in range(len(ant.path)-1):
row1, col1 = ant.path[i]
row2, col2 = ant.path[i+1]
pheromone[row1][col1] += Q / ((row2-row1)**2 + (col2-col1)**2)
# 更新最佳路径
best_path = None
best_distance = float('inf')
for ant in ants:
distance = sum(((ant.path[i][0]-ant.path[i+1][0])**2 + (ant.path[i][1]-ant.path[i+1][1])**2)**0.5 for i in range(len(ant.path)-1))
if distance < best_distance:
best_distance = distance
best_path = ant.path
# 输出最佳路径
print(best_path)
```
在搜索过程中,我们首先初始化信息素,并定义了一些参数,包括alpha、beta、Q、蚂蚁数量和迭代次数。然后,我们开始迭代搜索,每次迭代都用多个蚂蚁来搜索路径,并更新信息素。在每次迭代结束后,我们更新最佳路径。最后输出最佳路径即可。
完整代码如下:
相关推荐
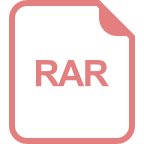














