esp32cam+opencv+python 实现数字识别
时间: 2023-07-28 16:07:20 浏览: 705
实现数字识别的整个过程可以分为以下几步:
1. 使用ESP32-CAM采集图像并通过WiFi将图像传输到计算机。
2. 在计算机上使用Python和OpenCV对图像进行处理,包括二值化、轮廓检测、字符分割等。
3. 对每个字符进行数字识别,可以使用深度学习算法(如卷积神经网络)或传统机器学习算法(如支持向量机)。
4. 将识别结果返回给ESP32-CAM,可以通过串口或WiFi等方式将结果传输回ESP32-CAM。
下面是一个简单的示例代码,演示如何使用ESP32-CAM、Python和OpenCV实现数字识别:
```python
import cv2
import numpy as np
import requests
import json
# ESP32-CAM的IP地址和端口号
ip = '192.168.1.100'
port = '80'
# 发送HTTP请求获取图像
url = 'http://' + ip + ':' + port + '/capture'
response = requests.get(url)
img_array = np.array(bytearray(response.content), dtype=np.uint8)
img = cv2.imdecode(img_array, cv2.IMREAD_COLOR)
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对图像进行二值化处理
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 对图像进行膨胀操作,使字符区域更加连通
kernel = np.ones((3, 3), np.uint8)
dilation = cv2.dilate(thresh, kernel, iterations=1)
# 查找图像中的轮廓
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 对每个轮廓进行字符分割和数字识别
digits = []
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if w > 10 and h > 10:
roi = thresh[y:y+h, x:x+w]
roi = cv2.resize(roi, (28, 28))
roi = roi.reshape((1, 28, 28, 1)).astype('float32') / 255.0
# 发送HTTP请求进行数字识别
data = json.dumps({'inputs': roi.tolist()})
headers = {'content-type': 'application/json'}
url = 'http://' + ip + ':' + port + '/predict'
response = requests.post(url, data=data, headers=headers)
result = json.loads(response.text)['outputs']
digit = np.argmax(result)
digits.append(digit)
# 将识别结果返回给ESP32-CAM
data = json.dumps({'digits': digits})
headers = {'content-type': 'application/json'}
url = 'http://' + ip + ':' + port + '/result'
response = requests.post(url, data=data, headers=headers)
```
在这个示例中,我们通过发送HTTP请求获取ESP32-CAM采集的图像,并在计算机上使用OpenCV对图像进行处理。我们首先将图像转换为灰度图像,然后对图像进行二值化处理,使字符区域变为黑色,背景变为白色。接着对图像进行膨胀操作,使字符区域更加连通。然后查找图像中的轮廓,对每个轮廓进行字符分割和数字识别。我们使用Keras框架训练了一个卷积神经网络模型,用于数字识别。最后将识别结果通过HTTP请求返回给ESP32-CAM。
阅读全文
相关推荐
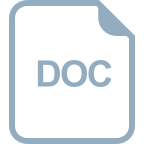
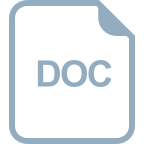
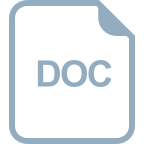
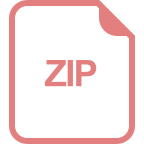
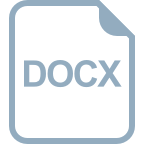
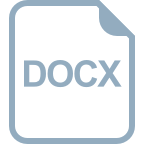
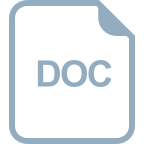
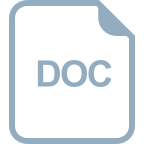






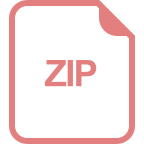
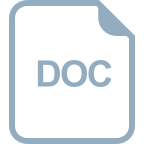
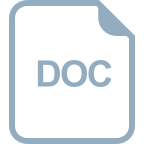

