链队列的基本操作代码c语言
时间: 2023-09-29 15:03:35 浏览: 46
链队列的基本操作包括:初始化、入队、出队、获取队头元素、判断队列是否为空、获取队列长度。
下面是链队列的基本操作的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 链队列结点
typedef struct QNode {
int data;
struct QNode *next;
} QNode;
// 队列结构体
typedef struct {
QNode *front; // 队头指针
QNode *rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = (QNode *) malloc(sizeof(QNode));
if (q->front == NULL) {
printf("初始化队列失败,内存分配失败!\n");
exit(-1);
}
q->front->next = NULL;
}
// 入队
void enQueue(Queue *q, int data) {
QNode *p = (QNode *) malloc(sizeof(QNode));
if (p == NULL) {
printf("入队失败,内存分配失败!\n");
exit(-1);
}
p->data = data;
p->next = NULL;
q->rear->next = p;
q->rear = p;
}
// 出队
void deQueue(Queue *q) {
if (q->front == q->rear) {
printf("出队失败,队列已空!\n");
exit(-1);
}
QNode *p = q->front->next;
q->front->next = p->next;
if (q->rear == p) q->rear = q->front;
free(p);
}
// 获取队头元素
int getFront(Queue *q) {
if (q->front == q->rear) {
printf("获取队头元素失败,队列已空!\n");
exit(-1);
}
QNode *p = q->front->next;
return p->data;
}
// 判断队列是否为空
int isEmpty(Queue *q) {
return q->front == q->rear;
}
// 获取队列长度
int getLength(Queue *q) {
int len = 0;
QNode *p = q->front->next;
while (p != NULL) {
len++;
p = p->next;
}
return len;
}
// 打印队列中的元素
void printQueue(Queue *q) {
QNode *p = q->front->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Queue q;
initQueue(&q);
// 入队
enQueue(&q, 1);
enQueue(&q, 2);
enQueue(&q, 3);
printf("队列中的元素为:");
printQueue(&q);
// 出队
deQueue(&q);
printf("出队后队列中的元素为:");
printQueue(&q);
// 获取队头元素
printf("队头元素为:%d\n", getFront(&q));
// 判断队列是否为空
printf("队列是否为空:%d\n", isEmpty(&q));
// 获取队列长度
printf("队列长度为:%d\n", getLength(&q));
return 0;
}
```
相关推荐
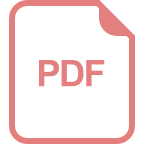
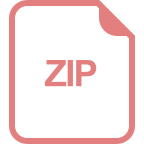
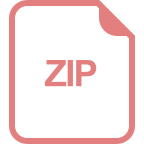
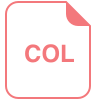














