implement a resource pool with C++
时间: 2024-04-10 14:26:39 浏览: 102
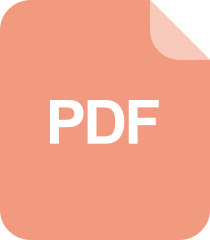
Visual.C++.MFC编程实例
To implement a resource pool in C++, you can use the concept of object pooling. Object pooling is a design pattern that allows you to reuse objects instead of creating and destroying them frequently, which can be expensive in terms of memory allocation and deallocation.
Here's a basic example of how you can implement a resource pool in C++:
```cpp
#include <queue>
#include <mutex>
#include <condition_variable>
template<typename T>
class ResourcePool {
private:
std::queue<T*> pool;
std::mutex mutex;
std::condition_variable condition;
public:
ResourcePool(int initialSize) {
for (int i = 0; i < initialSize; ++i) {
pool.push(new T());
}
}
~ResourcePool() {
while (!pool.empty()) {
delete pool.front();
pool.pop();
}
}
T* acquireResource() {
std::unique_lock<std::mutex> lock(mutex);
if (pool.empty()) {
// Wait until a resource is available
condition.wait(lock);
}
T* resource = pool.front();
pool.pop();
return resource;
}
void releaseResource(T* resource) {
std::unique_lock<std::mutex> lock(mutex);
pool.push(resource);
// Notify waiting threads that a resource is available
condition.notify_one();
}
};
```
In this example, the `ResourcePool` class uses a queue to store the resources. The `acquireResource` function is responsible for acquiring a resource from the pool. If the pool is empty, it waits until a resource becomes available using the `std::condition_variable`. The `releaseResource` function is used to release a resource back into the pool.
You can customize this implementation based on your specific requirements. For example, you can add additional functions to initialize or resize the pool dynamically.
阅读全文
相关推荐
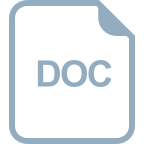
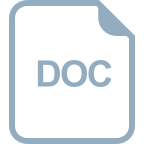



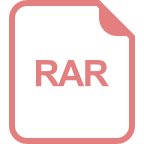
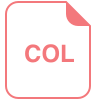
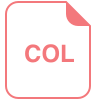
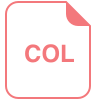
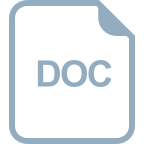
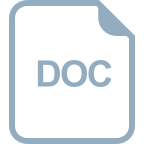
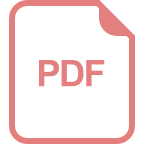
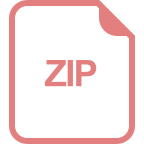
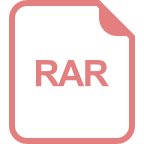
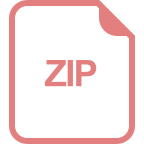
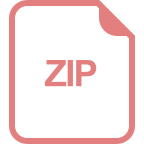
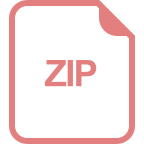
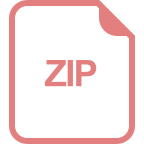