implement a shared resource pool in C++
时间: 2024-04-15 09:27:32 浏览: 9
To implement a shared resource pool in C++, you can modify the previous example by introducing shared ownership of resources using smart pointers. Here's an updated version:
```cpp
#include <iostream>
#include <queue>
#include <mutex>
#include <condition_variable>
#include <memory>
template<typename T>
class ResourcePool {
public:
ResourcePool(size_t maxSize) : max_size(maxSize), available_resources(0) {}
std::shared_ptr<T> acquire() {
std::unique_lock<std::mutex> lock(mutex);
while (resources.empty()) {
if (available_resources >= max_size) {
// Wait until a resource is released
cv.wait(lock);
} else {
// Create a new resource if the pool is not full
resources.push(createResource());
++available_resources;
}
}
std::shared_ptr<T> resource = resources.front();
resources.pop();
return resource;
}
void release(std::shared_ptr<T> resource) {
std::lock_guard<std::mutex> lock(mutex);
resources.push(resource);
cv.notify_one();
}
private:
size_t max_size;
size_t available_resources;
std::queue<std::shared_ptr<T>> resources;
std::mutex mutex;
std::condition_variable cv;
// Create a new resource object (replace with your own code)
std::shared_ptr<T> createResource() {
return std::make_shared<T>();
}
};
// Example usage
class MyResource {
public:
void use() {
std::cout << "Using resource" << std::endl;
}
};
int main() {
ResourcePool<MyResource> pool(5);
// Acquire and use resources
for (int i = 0; i < 10; ++i) {
std::shared_ptr<MyResource> resource = pool.acquire();
resource->use();
pool.release(resource);
}
return 0;
}
```
In this updated example, the `ResourcePool` class now uses `std::shared_ptr` to manage shared ownership of resources. When acquiring a resource, a `std::shared_ptr` is returned instead of a raw pointer. This allows multiple threads or parts of the code to safely share and use the resource without worrying about premature destruction.
When releasing a resource, a `std::shared_ptr` is passed back to the pool instead of a raw pointer.
Note that the `createResource` function now uses `std::make_shared` to create the resource object, which ensures that the object is properly managed by a shared pointer.
By using shared pointers, you can safely share and manage resources within the resource pool, allowing multiple parts of your code to use them without concerns about memory ownership and deallocation.
相关推荐
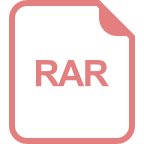
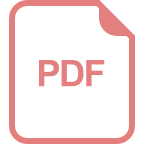














