implement a resource pool in C++
时间: 2024-04-13 08:29:17 浏览: 20
To implement a resource pool in C++, you can use a combination of data structures and synchronization mechanisms. Here's a basic outline of how you can approach it:
1. Define a class to represent the resource pool. This class will have member variables to hold the resources and any necessary data structures for managing them.
2. Initialize the resource pool with a fixed number of resources. You can use a container, such as a vector or an array, to store the resources.
3. Implement a method to acquire a resource from the pool. This method will handle synchronization to ensure that multiple threads can safely access the pool. You can use a mutex or a semaphore to achieve this.
4. When a resource is acquired, mark it as "in use" so that other threads cannot access it concurrently.
5. Implement a method to release a resource back to the pool. This method will also handle synchronization to ensure that the resource is correctly returned.
6. When a resource is released, mark it as "available" so that it can be acquired by other threads.
Here's a simplified example to illustrate the concept:
```cpp
#include <vector>
#include <mutex>
class ResourcePool {
public:
ResourcePool(int numResources) : resources(numResources, true) {}
int acquireResource() {
std::unique_lock<std::mutex> lock(mutex);
for (int i = 0; i < resources.size(); ++i) {
if (resources[i]) {
resources[i] = false;
return i;
}
}
return -1; // No available resources
}
void releaseResource(int index) {
std::unique_lock<std::mutex> lock(mutex);
if (index >= 0 && index < resources.size()) {
resources[index] = true;
}
}
private:
std::vector<bool> resources;
std::mutex mutex;
};
int main() {
ResourcePool pool(5);
// Example usage
int resourceIndex = pool.acquireResource();
if (resourceIndex != -1) {
// Use the resource
// ...
pool.releaseResource(resourceIndex);
}
return 0;
}
```
Please note that this example provides a basic framework for a resource pool and may require further modifications or enhancements based on your specific requirements.
相关推荐
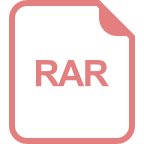
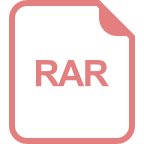
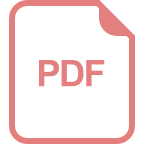














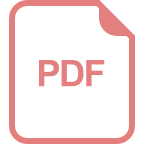