利用Python提取//*[@id="wrapper"]/div[5]/div[2]中的文字
时间: 2024-01-26 12:02:55 浏览: 20
你可以使用 Python 的 requests 和 lxml 库来提取网页中的元素。以下是一个例子:
```python
import requests
from lxml import etree
url = 'http://www.example.com' # 将URL替换为你要提取的网页
response = requests.get(url)
html = etree.HTML(response.content)
text = html.xpath('//*[@id="wrapper"]/div[5]/div[2]//text()')
print(text)
```
上面的代码会发送一个 GET 请求到指定的 URL,然后使用 lxml 库的 etree.HTML() 方法将响应内容转换成 HTML 对象。接着,使用 xpath() 方法提取指定元素的文字。在这个例子中,我们提取了 ID 为 "wrapper" 的元素下第 5 个子元素下的第 2 个子元素的所有文字。最后,将提取到的文字打印出来。
相关问题
def parse(self, response): res = Selector(response) items = RenrenchesipderItem() items['id'] = res.xpath('//div[@class="detail-wrapper"]/@data-encrypt-id').extract()[0] # 标题 items['title'] = res.xpath('//div[@class="title"]/h1/text()').extract()[0] # 客户出价 items['price'] = res.xpath('//div[@class="middle-content"]/div/p[2]/text()').extract()[0] # 市场价 items['new_car_price'] = res.xpath('//div[@class="middle-content"]/div/div[1]/span/text()').extract()[0] # 首付款 down_payment = res.xpath('//div[@class="list"]/p[@class="money detail-title-right-tagP"]/text()') # 月供 monthly_payment = res.xpath('//[@id="basic"]/div[2]/div[2]/div[1]/div[3]/div[2]/p[5]/text()') # 判断是否可以分期购买 if down_payment and monthly_payment: items['staging_info'] = [down_payment.extract()[0], monthly_payment.extract()[0]] # 服务费 items['service_fee'] = res.xpath('///div[1]/p[2]/strong/text()').extract()[0] # 服务项 items['service'] = res.xpath('//[@id="js-box-service"]/table/tr/td/table/tr/td/text()').extract() # 车辆上牌时间 里程 外迁信息 items['info'] = res.xpath('///div[2]/div[2]/div[1]/div[4]/ul/li/div/p/strong/text()').extract() # 车辆排量 items['displacement'] = \ res.xpath('//[@id="basic"]/div[2]/div[2]/div[1]/div[4]/ul/li[4]/div/strong/text()').extract()[0] # 车辆上牌城市 items['registration_city'] = res.xpath('///@licensed-city').extract()[0] # 车源号 items['options'] = \ res.xpath('//*[@id="basic"]/div[2]/div[2]/div[1]/div[5]/p/text()').extract()[0].strip().split(":")[1] # 判断是都有图片 if res.xpath('//div[@class="info-recommend"]/div/img/@src'): # 车辆图片 items['car_img'] = res.xpath('//div[@class="info-recommend"]/div/img/@src').extract()[0] # 车辆所在城市 items['city'] = res.xpath('//div[@rrc-event-scope="city"]/a[@class="choose-city"]/text()').extract()[0].strip() # 车辆颜色 items['color'] = res.xpath('//div[@class="card-table"]/table/tr/td[2]/text()').extract()[0] 解释一下每一句话
这是一个 Python 的 scrapy 爬虫程序中的一个解析函数,主要作用是从网页中提取出需要的数据并存储到 items 中。具体解释如下:
```python
def parse(self, response):
res = Selector(response) # 使用 scrapy 的 Selector 对象解析 response
items = RenrenchesipderItem() # 定义一个 items 对象用于存储数据
# 从网页中提取出 id、标题、客户出价、市场价、首付款、月供、服务费、服务项、车辆上牌时间、里程、外迁信息、车辆排量、车辆上牌城市、车源号、车辆颜色等数据
items['id'] = res.xpath('//div[@class="detail-wrapper"]/@data-encrypt-id').extract()[0]
items['title'] = res.xpath('//div[@class="title"]/h1/text()').extract()[0]
items['price'] = res.xpath('//div[@class="middle-content"]/div/p[2]/text()').extract()[0]
items['new_car_price'] = res.xpath('//div[@class="middle-content"]/div/div[1]/span/text()').extract()[0]
down_payment = res.xpath('//div[@class="list"]/p[@class="money detail-title-right-tagP"]/text()')
monthly_payment = res.xpath('//[@id="basic"]/div[2]/div[2]/div[1]/div[3]/div[2]/p[5]/text()')
if down_payment and monthly_payment:
items['staging_info'] = [down_payment.extract()[0], monthly_payment.extract()[0]]
items['service_fee'] = res.xpath('///div[1]/p[2]/strong/text()').extract()[0]
items['service'] = res.xpath('//[@id="js-box-service"]/table/tr/td/table/tr/td/text()').extract()
items['info'] = res.xpath('///div[2]/div[2]/div[1]/div[4]/ul/li/div/p/strong/text()').extract()
items['displacement'] = res.xpath('//[@id="basic"]/div[2]/div[2]/div[1]/div[4]/ul/li[4]/div/strong/text()').extract()[0]
items['registration_city'] = res.xpath('///@licensed-city').extract()[0]
items['options'] = res.xpath('//*[@id="basic"]/div[2]/div[2]/div[1]/div[5]/p/text()').extract()[0].strip().split(":")[1]
if res.xpath('//div[@class="info-recommend"]/div/img/@src'):
items['car_img'] = res.xpath('//div[@class="info-recommend"]/div/img/@src').extract()[0]
items['city'] = res.xpath('//div[@rrc-event-scope="city"]/a[@class="choose-city"]/text()').extract()[0].strip()
items['color'] = res.xpath('//div[@class="card-table"]/table/tr/td[2]/text()').extract()[0]
return items # 返回 items 对象
```
其中,Selector 对象是 scrapy 中提供的一种选择器,可以方便地从 HTML 或 XML 中提取数据。extract() 方法用于获取提取的数据,[0] 则是因为返回的是一个列表,需要取出其中的第一个元素。此外,还有一些判断语句用于处理数据缺失或判断是否存在该数据,如 if down_payment and monthly_payment: 判断是否可以分期购买。最后,将所有数据存储在 items 对象中并返回。
使用python获取http://college.gaokao.com/schlist/p当中的学校名和高校所在地
您好!针对您的问题,我可以提供以下Python代码来获取http://college.gaokao.com/schlist/p中的学校名和高校所在地,具体如下:
```python
import requests
from bs4 import BeautifulSoup
url = "http://college.gaokao.com/schlist/p"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
for item in soup.select("ul#wrapper li"):
name = item.select_one("h2 a").text.strip()
location = item.select_one("div p a").text.strip()
print("学校名:{},高校所在地:{}".format(name, location))
```
该代码使用requests库实现对网页的请求,使用BeautifulSoup库对网页进行解析,然后通过CSS选择器获取每个学校的名称和所在地。最后使用Python的print函数将结果输出。
希望这个代码可以帮到您!
相关推荐
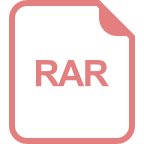
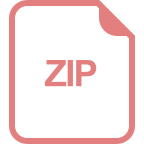
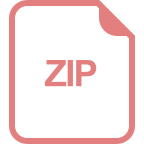












