HBase BulkLoad批量写入数据代码
时间: 2024-05-10 13:21:34 浏览: 127
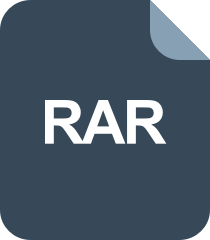
jobs_hbase_mirrorm5y_BulkLoadjava_
以下是一个简单的HBase BulkLoad批量写入数据代码示例:
```java
Configuration config = HBaseConfiguration.create();
config.set("hbase.zookeeper.quorum", "localhost"); // HBase ZooKeeper Quorum
config.set("hbase.zookeeper.property.clientPort", "2181"); // HBase ZooKeeper Client端口
String tableName = "mytable";
Path inputPath = new Path("/path/to/input/file");
Path outputPath = new Path("/path/to/output/file");
// 创建HBase表
HBaseAdmin admin = new HBaseAdmin(config);
HTableDescriptor tableDesc = new HTableDescriptor(tableName);
tableDesc.addFamily(new HColumnDescriptor("cf"));
admin.createTable(tableDesc);
// 配置BulkLoad
BulkLoadHelper helper = new BulkLoadHelper(config);
helper.setHTable(new HTable(config, tableName));
helper.setInputPath(inputPath);
helper.setOutputPath(outputPath);
helper.setBulkLoadDir(new Path("/path/to/bulkload/directory"));
helper.setMapperClass(MyMapper.class);
// 运行BulkLoad
int result = helper.run();
// 关闭Admin和HTable
admin.close();
helper.getHTable().close();
```
上述代码中,`MyMapper`是自定义的Mapper类,用于将输入文件中的数据转换为HBase表中的Put操作。以下是一个简单的`MyMapper`类示例:
```java
public static class MyMapper extends Mapper<LongWritable, Text, ImmutableBytesWritable, Put> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
// 将第一个字段作为HBase行键
byte[] rowKey = Bytes.toBytes(fields[0]);
ImmutableBytesWritable key = new ImmutableBytesWritable(rowKey);
// 创建HBase Put操作
Put put = new Put(rowKey);
put.addColumn(Bytes.toBytes("cf"), Bytes.toBytes("col1"), Bytes.toBytes(fields[1]));
put.addColumn(Bytes.toBytes("cf"), Bytes.toBytes("col2"), Bytes.toBytes(fields[2]));
context.write(key, put);
}
}
```
`MyMapper`类的作用是将输入文件中的每行数据转换为一个HBase表中的Put操作。在这个例子中,我们假设输入文件中的每行数据都包含三个字段,第一个字段作为HBase表中的行键,第二个和第三个字段作为HBase表中的列。因此,我们将第一个字段作为HBase Put操作的行键,将第二个和第三个字段作为HBase Put操作的列。最后,我们将每个Put操作的行键和操作本身作为输出传递给Hadoop MapReduce框架。
阅读全文
相关推荐
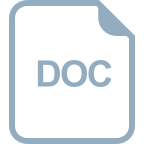
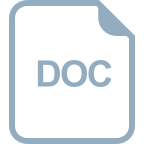

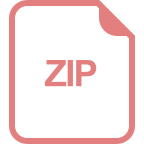
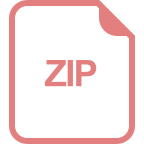
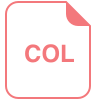
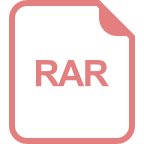
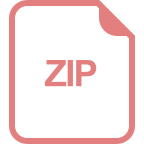
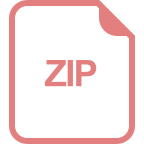
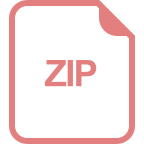
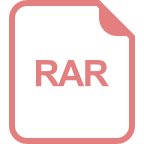
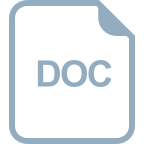
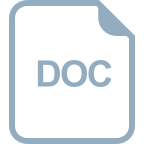
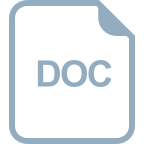
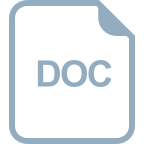
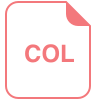

